Are you still zipping files manually or juggling clunky third-party libraries? There’s a better way right inside your .NET toolbox! In this deep dive, I’ll show you how to become a true compression wizard using C#’s built-in ZipArchive
class. Whether you’re packaging logs, bundling uploads, or automating backups, you’ll walk away with clear, production-ready techniques. Ready? Let’s zip through it!
Understanding ZipArchive in C#
What is ZipArchive?
ZipArchive
is part of the System.IO.Compression
namespace, giving .NET developers a powerful tool to work with ZIP files natively. No need for external dependencies! Introduced with .NET Framework 4.5 and enhanced across .NET Core and .NET 5+, it’s a reliable choice for both legacy and modern applications.
- Purpose: Create, update, read, and extract ZIP archives.
- Compatibility: Works seamlessly with .NET Framework 4.5+, .NET Core, and .NET 5/6/7.
- Bonus: It’s part of BCL (Base Class Library), meaning performance and updates are handled by Microsoft.
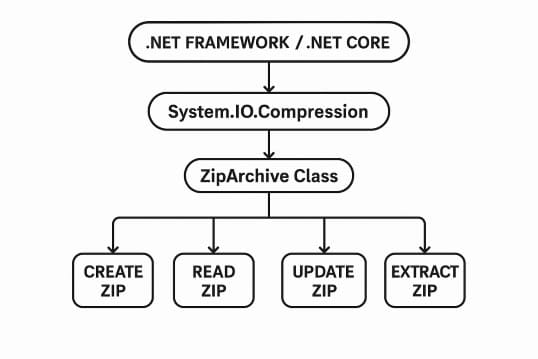
Why Use ZipArchive?
- Built-in convenience: No third-party libraries needed.
- Strong performance: Optimized for speed and memory usage.
- Cross-platform: Windows, Linux, macOS.
- Ease of use: Clean API and simple integration.
I’ve used ZipArchive
in many enterprise apps for nightly backups and export features. It’s robust and keeps your stack clean.
Setting Up Your Project
Installing Required Packages
In .NET Core and .NET 5+, System.IO.Compression
is built-in. But for older .NET Framework apps, you might need:
Install-Package System.IO.Compression
Install-Package System.IO.Compression.FileSystem
Namespaces to Include
Add these at the top of your file:
using System.IO;
using System.IO.Compression;
Simple as that, you’re ready to zip and unzip!
Creating ZIP Files in C#
Basic Example: Zipping Files
Let’s start with a straightforward example:
using (ZipArchive archive = ZipFile.Open("archive.zip", ZipArchiveMode.Create))
{
archive.CreateEntryFromFile(@"C:\\path\\to\\file.txt", "file.txt");
}
Explanation:
ZipFile.Open
: Opens or creates a ZIP file.ZipArchiveMode.Create
: Creates a new archive or overwrites.CreateEntryFromFile
: Adds a file to the ZIP.
Adding Files Dynamically
Say you want to add files generated at runtime:
var files = Directory.GetFiles(@"C:\\path\\to\\folder");
using (ZipArchive archive = ZipFile.Open("dynamic.zip", ZipArchiveMode.Create))
{
foreach (var file in files)
{
archive.CreateEntryFromFile(file, Path.GetFileName(file));
}
}
Tip: Always use Path.GetFileName()
to avoid nesting unwanted folders.
Setting Compression Levels
Compression levels can make a big difference:
archive.CreateEntryFromFile(file, Path.GetFileName(file), CompressionLevel.Optimal);
Optimal
: Best size, slower.Fastest
: Bigger files, faster.NoCompression
: No compression, just packaging.
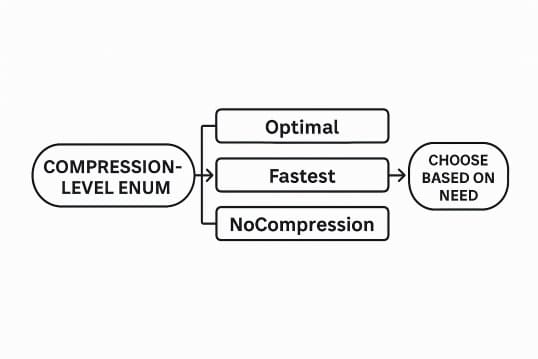
Extracting ZIP Files
Extracting All Files from a ZIP
ZipFile.ExtractToDirectory("archive.zip", @"C:\\extracted");
Done. That simple!
Extracting Specific Files
using (ZipArchive archive = ZipFile.OpenRead("archive.zip"))
{
var entry = archive.GetEntry("file.txt");
entry?.ExtractToFile(@"C:\\extracted\\file.txt");
}
Tip: Always check for null
entries.
Handling Extraction Errors
Wrap your extraction logic with try-catch to handle corrupt files or permission issues:
try
{
ZipFile.ExtractToDirectory("archive.zip", @"C:\\extracted");
}
catch (InvalidDataException ex)
{
Console.WriteLine($"Corrupt archive: {ex.Message}");
}
catch (IOException ex)
{
Console.WriteLine($"IO Error: {ex.Message}");
}
Advanced Techniques
Working with In-Memory Streams
using (var memoryStream = new MemoryStream())
{
using (var archive = new ZipArchive(memoryStream, ZipArchiveMode.Create, true))
{
var entry = archive.CreateEntry("inmemory.txt");
using (var entryStream = entry.Open())
using (var streamWriter = new StreamWriter(entryStream))
{
streamWriter.Write("Hello, In-Memory ZIP!");
}
}
File.WriteAllBytes("inmemory.zip", memoryStream.ToArray());
}
Modifying Existing ZIP Files
using (var archive = ZipFile.Open("archive.zip", ZipArchiveMode.Update))
{
archive.CreateEntryFromFile(@"C:\\newfile.txt", "newfile.txt");
}
Reading ZIP Contents Without Extracting
using (var archive = ZipFile.OpenRead("archive.zip"))
{
foreach (var entry in archive.Entries)
{
Console.WriteLine($"{entry.FullName} - {entry.Length} bytes");
}
}
Best Practices and Performance Tips
Streamlining File I/O Operations
- Use
using
statements to manage streams and archives. - For large files, process streams directly to minimize memory usage.
- Avoid loading entire files into memory when streaming is possible.
Security Considerations
Watch out for Zip Slip vulnerabilities!
var fullPath = Path.GetFullPath(Path.Combine(extractionPath, entry.FullName));
if (!fullPath.StartsWith(extractionPath, StringComparison.OrdinalIgnoreCase))
{
throw new UnauthorizedAccessException("Zip entry is outside of the target dir!");
}
FAQ: Common Questions About C# ZipArchive
Absolutely! Just include directory separators in the entry name: archive.CreateEntry("folder/subfolder/file.txt");
Not natively. Consider third-party libraries if you need encryption.
Yes! Open in ZipArchiveMode.Update
, remove the old entry, and add the new one.
Stream entries to avoid memory overload, and consider splitting archives if needed.
Conclusion: Zip Like a Pro in C#
With ZipArchive
, you hold a mighty tool in your .NET arsenal! Whether you’re building CI/CD pipelines, creating automated backups, or streamlining file exchanges, these techniques will make your C# applications leaner and meaner.
So, what will you zip first? Share your use case in the comments or explore other powerful .NET I/O capabilities on the blog. Let’s keep learning and building together!