DotNetFiddle is a cutting-edge online platform that transforms the way developers work with C#. It offers a smooth and intuitive environment for coding, testing, and sharing C# code, making it an essential resource for both novices and seasoned programmers. This guide aims to familiarize you with DotNetFiddle, providing an overview of its features, capabilities, and how to leverage it to improve your C# programming skills. Whether you’re just starting out in programming or seeking to polish your expertise, this guide is your gateway to discovering the full potential of DotNetFiddle.
What is DotNetFiddle?
DotNetFiddle is an online Integrated Development Environment (IDE) designed for C#, F#, and VB.NET. It allows users to write, test, and share code snippets or entire applications in a cloud-based setting.
Key features include:
- Code Execution: Instantly run your C# code without needing a local setup.
- Multiple Language Support: Choose between C#, F#, and VB.NET.
- Integrated NuGet Package Manager: Easily include external libraries and frameworks in your projects.
- Real-time Collaboration: Share your code with others and work together in real time.
- Pre-configured Templates: Kickstart your coding with a variety of templates.
DotNetFiddle is a beginner-friendly platform, making it simple to experiment with C# code, grasp core concepts, and collaborate with others in the programming community.
Getting Started with DotNetFiddle
To get started with Dot Net Fiddle, simply navigate to the website at dotnetfiddle.net. You’ll be presented with a blank code editor and a few options for selecting the version of .NET you’d like to use and the type of project you’d like to create.
To start using Dot Net Fiddle, follow these simple steps:
- Go to the website at dotnetfiddle.net.
- After opening website, you will be presented with a blank code editor and several options for selecting the version of .NET that you want to use and the type of project that you want to create.
- Choose the version of .NET that you want to use. You can select from various versions of .NET (.NET Core, .NET Framework, and .NET Standard). Depending on your choice, the options for the type of project you can create may vary.
- Select the type of project that you want to create. You can create console apps, web apps, class libraries, and more. This will determine the starting code that you will see in the editor.
- Now you can start writing your code. The editor is similar to other code editors you may be familiar with, and includes features like syntax highlighting, code completion, and error highlighting.
- After it, you can compile and run it using the buttons at the top of the editor. The “Run” button will compile and execute your code, and the output will be displayed in a panel below the editor.
- One of the most useful features of DotNetFiddle is the ability to share code snippets with others. To share your code, simply copy the URL from your browser’s address bar and send it to whomever you’d like to share it with.
Writing and Running Code
Let’s start by writing a simple “Hello, Dot Net Fiddle!” program:
using System;
public class Program
{
public static void Main()
{
Console.WriteLine("Hey, Dot Net Fiddle!");
}
}
- Coding Area: The main coding area is where you write your C# code. You can create classes, methods, and other code structures just like you would in a traditional IDE.
- Run Button: Click the
"Run"
button (green arrow) to compile and execute your code. The output will be displayed in the"Output"
panel below the coding area.
Managing NuGet Packages
DotNetFiddle allows you to manage NuGet packages to include external libraries and dependencies in your code.
Let’s use the `Newtonsoft.Json
` library as an example. We’ll deserialize a JSON string:
using System;
using Newtonsoft.Json;
public class Program
{
public static void Main()
{
string json = "{\"personName\":\"Alex\",\"personAge\":39}";
Person individual = JsonConvert.DeserializeObject<Person>(json);
Console.WriteLine($"Name: {individual.PersonName}, Age: {individual.PersonAge}");
}
}
public class Person
{
public string PersonName { get; set; }
public int PersonAge { get; set; }
}
- NuGet Panel: Click the
"Add NuGet Package"
button on the left-hand panel to search for and add NuGet packages. For our example we need"Newtonsoft.Json"
. Search and select it and click the"Add"
button.
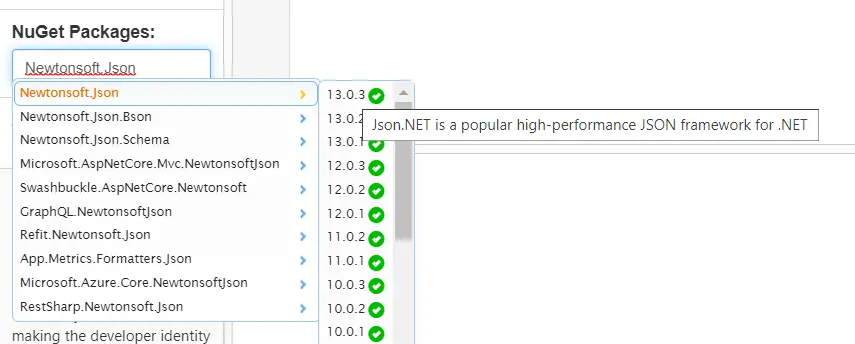
- Package Configuration: After adding the package, you can reference the required namespaces and use the library’s functionality in your code.
See this example at DotNetFiddle: Deserialize Object
Sharing and Collaboration
.NET Fiddle makes it easy to share your code snippets and collaborate with others.
- Share Button: Click the
"Share"
button to generate a URL that anyone can use to access your code snippet. You can set the visibility to"Public"
or"Only With Link"
. - Collaboration: Multiple users can collaborate on a shared snippet in real-time. Just share the URL, and everyone can edit and see changes as they happen.
Saving Snippets
You can save your code snippets directly on .NET Fiddle for future reference or sharing.
Save Button: Click the "Save"
button to create an account or log in if you haven’t already. After logging in, you can save your snippet with a title and optional description.
Tips and Tricks
- Auto-Complete: .NET Fiddle offers a robust auto-complete feature. As you type, it will suggest relevant methods and properties, speeding up your coding process.
- Code Tidying: Use the
"TidyUp"
button to automatically format your code, making it more readable. - Console Interaction: You can read input from the console using
Console.ReadLine()
, making it possible to create interactive snippets.
DotNetFiddle is a powerful online code editor designed to make writing, testing, and sharing C# code snippets a breeze. It boasts features such as live execution, NuGet package management, and collaboration tools, among others. Whether you’re just starting out with C# or you’re a seasoned developer working on new ideas, DotNetFiddle is an essential resource.
Take the time to explore its wide range of features, try out different code snippets, and fully utilize the platform.
Happy coding!
FAQ
.NET Fiddle is an online tool designed for developers to write, compile, and test .NET code snippets without the need for local software installations. It is specifically tailored to the .NET framework and offers support for multiple programming languages, such as C#, VB.NET, and F#. This platform streamlines the development process, allowing for efficient coding and testing in a convenient, web-based environment.
While there are many online code editors, .NET Fiddle is specifically designed for the .NET framework, making it unique. It also offers features like adding NuGet packages directly within the platform.
You can access .NET Fiddle by visiting https://dotnetfiddle.net/. Once there, you’ll be presented with an editor to start coding immediately.
After entering your code into the editor, simply click the “Run” button located at the top of the page. The output will be displayed below the editor.
Yes, .NET Fiddle allows you to add NuGet packages to your projects. Click on the “NuGet Packages” tab, search for the desired package, and click “Add”.
Once you’ve created your code snippet, click on the “Share” button at the top of the page. This will generate a unique URL that you can share with others.
Yes, .NET Fiddle offers a robust auto-complete feature that suggests relevant methods and properties as you type, helping to speed up the coding process.
Absolutely! Use the “TidyUp” button to automatically format your code, enhancing its readability.
Yes, you can read input from the console using Console.ReadLine()
, allowing you to create interactive code snippets.
.NET Fiddle is a free platform, making it accessible to everyone who wishes to write, compile, and test .NET code online.
Hey, simple question… How do I see errors in my code?
So, errors are highlighted in the code editor, and you can also see them in the output panel when you run your code.
DotNetFiddle looks like a powerful tool. Thanks for breaking down its features in such an accessible way!
I am trying to use fiddle in c# but it deletes characters immediately after typing. So for example it wont let me type private, since as soon as I type p it deletes it. What am I missing?
Experiencing character deletion in DotNetFiddle could be due to outdated browsers or interference from browser extensions like ad blockers. Ensure your browser is updated, disable extensions, and clear cache/cookies to see if it resolves the issue.
So If problems persist, check for JavaScript errors via the console (F12) and consider reporting this behavior on DotNetFiddle’s forums with your browser details and steps to replicate the issue for further assistance.