Are you tired of constantly extending classes just to squeeze in a couple of helper methods? Or maybe you dream of enhancing existing types without the headache of inheritance? Well, let me tell you: extension methods in C# feel like a developer’s “cheat code” for cleaner, more expressive code. Today, we’re going to unpack this powerful feature that can supercharge your development workflow.
What Are Extension Methods?
Simply put, extension methods let you “add” methods to existing types without modifying their source code or using inheritance. Think of them as a way to gift existing classes with new superpowers while keeping your code organized.
They live inside static classes, and they make your code more expressive and fluent. You often see them in action with LINQ, like .Select()
and .Where()
. But there’s much more you can do!
Why Not Inheritance?
Inheritance is great, but:
- It tightly couples your classes.
- It clutters hierarchies when all you need is an extra method or two.
- It doesn’t work for sealed classes (like
String
orDateTime
).
Extension methods sidestep all these issues, giving you the flexibility of helpers with the elegance of natural syntax.
Anatomy of an Extension Method
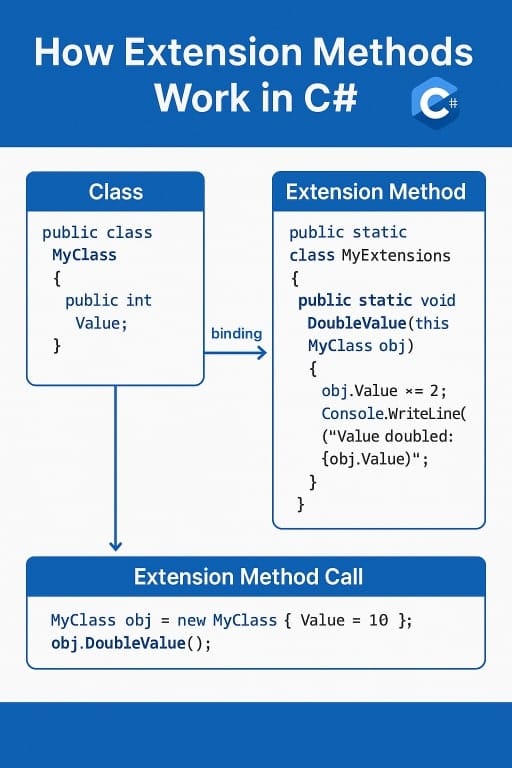
Here’s the recipe:
- Static class: The method must live in a static container.
- Static method: The method itself must be static.
- First parameter with
this
modifier: This tells the compiler you’re extending a type.
Example:
public static class StringExtensions
{
public static bool IsNullOrEmpty(this string input)
{
return string.IsNullOrEmpty(input);
}
}
// Usage
string name = null;
bool result = name.IsNullOrEmpty();
Explanation: Here, we added IsNullOrEmpty()
directly to string
. It reads naturally, feels like it’s part of the original class, and improves readability.
Real-World Use Cases
- Data validation: Add methods like
IsValidEmail()
to strings. - Collection utilities: Enhance
IEnumerable<T>
with custom filters. - Fluent APIs: Build chainable commands for better developer experience.
Example:
public static IEnumerable<T> NotNull<T>(this IEnumerable<T> source)
{
return source.Where(item => item != null);
}
// Usage
var cleanedList = myList.NotNull().ToList();
This removes null values from any list, making your data processing pipeline cleaner and safer.
Best Practices & Pitfalls
When to Use:
- When you need to extend types you don’t own.
- To improve readability and maintainability.
When to Avoid:
- When you already control the class (just add the method directly).
- Avoid overusing them – too many extensions can clutter IntelliSense.
Common Mistakes:
- Forgetting the
this
keyword. - Creating ambiguous method names.
Comparisons: Extension Methods vs. Alternatives
Approach | Pros | Cons |
---|---|---|
Extension Methods | Clean syntax, works with sealed classes | Can clutter IntelliSense |
Inheritance | Good for structural hierarchy | Overkill for utility methods |
Helper Classes | Simple to implement | Less natural syntax (e.g., Helper.DoSomething(x) ) |
Partial Classes | Good for organizing your own types | Works only within the same assembly |
Advanced Scenarios
Chaining Methods
public static string TrimAndLower(this string input)
{
return input.Trim().ToLower();
}
var result = " Hello World ".TrimAndLower();
Generic Extension Methods
public static bool IsDefault<T>(this T value)
{
return EqualityComparer<T>.Default.Equals(value, default(T));
}
LINQ-style Fluent Interfaces
Extension methods let you build pipelines of operations, making your code expressive and easy to read.
var processed = data
.NotNull()
.Where(x => x.IsActive)
.Select(x => x.Name);
Performance Considerations
- Compilation: Extension methods compile down to static method calls — no runtime magic.
- Runtime: Virtually no performance penalty. But don’t abuse them for everything!
Future-Proofing Your Code
C# keeps evolving, and extension methods are only getting better:
- C# 8.0: Nullable reference types improve extension method safety.
- C# 10.0+: More concise syntax and global
using
directives reduce boilerplate.
Keep an eye on modern patterns to keep your codebase sharp.
FAQ: Common Questions about Extension Methods
No, extension methods do not override; they act as syntactic sugar for static method calls.
Absolutely! This is a great way to extend behavior for multiple implementations.
Yes, but if you overdo it, IntelliSense can become crowded.
Conclusion: Supercharge Your Code with Extension Methods
As we’ve explored, extension methods are like secret power-ups for your C# toolkit. They keep your code clean, expressive, and flexible, all without the baggage of inheritance or bloated helper classes.
Now it’s your turn: experiment with extension methods in your projects! Trust me, once you start, you’ll wonder how you ever coded without them.
What’s the cleverest extension method you’ve written? Share your favorite use case in the comments below! And while you’re at it, explore open-source libraries like MoreLINQ for inspiration.