Are you still tying your methods directly to events like it’s 2005? Discover the secret sauce behind modern, clean, and scalable C# apps — it’s all about mastering delegates!
Ever felt like your code is becoming a tangled web of direct method calls and rigid dependencies? What if I told you there’s a way to loosen those chains and give your app wings of flexibility? Enter delegates in C#. They’re the unsung heroes of event-driven programming, quietly orchestrating method calls behind the scenes.
What Are Delegates in C#?
Definition and Basics
Let me break it down simply: delegates in C# are like function pointers in C or C++. They allow you to reference methods as variables. Imagine having a remote control where you can assign any command to a button. That’s a delegate — it points to methods you can invoke later.
- Delegate: Type-safe function pointer.
- Purpose: Store references to methods, invoke them dynamically.
- Analogy: Like hiring an assistant (delegate) to run tasks on your behalf.
Why Use Delegates?
Delegates bring power and flexibility to your applications. Here’s why you should care:
- Loose Coupling: Components don’t need to know about each other directly.
- Flexibility: Methods can be assigned at runtime.
- Essential for Events: Delegates are the backbone of event-driven programming in .NET.
In my experience, understanding delegates was a game-changer. It made my codebase cleaner and more modular, especially in large enterprise applications.
Anatomy of a Delegate
Declaring Delegates
public delegate void Notify(string message);
This defines a delegate that points to methods with a void
return type and a single string
parameter.
Explanation: You’re telling the compiler: “I need a method reference that fits this signature.”
Instantiating Delegates
public void ShowMessage(string message) => Console.WriteLine(message);
Notify notifier = new Notify(ShowMessage);
Explanation: We’ve linked the ShowMessage
method to the notifier
delegate instance.
Invoking Delegates
notifier("Hello from the delegate!");
Explanation: Just like calling a method, but through the delegate reference!
Delegate Types and Use Cases
Singlecast Delegates
Notify singleNotifier = ShowMessage;
singleNotifier("Singlecast delegate in action!");
Singlecast delegates reference a single method. Simple and efficient.
Multicast Delegates
Notify multiNotifier = ShowMessage;
multiNotifier += (msg) => Console.WriteLine("Log: " + msg);
multiNotifier("Multicast example");
Multicast delegates can point to multiple methods. All subscribed methods will execute in order.
Anonymous Methods and Lambda Expressions
Notify lambdaNotifier = delegate (string msg) {
Console.WriteLine("Anonymous: " + msg);
};
Notify shortLambda = (msg) => Console.WriteLine("Lambda: " + msg);
Use case: When you need quick, inline methods without formal declarations.
Delegates in Event-Driven Programming
Understanding Events and Delegates
Delegates are foundational to events in .NET. When you declare an event, you’re essentially using a delegate behind the scenes.
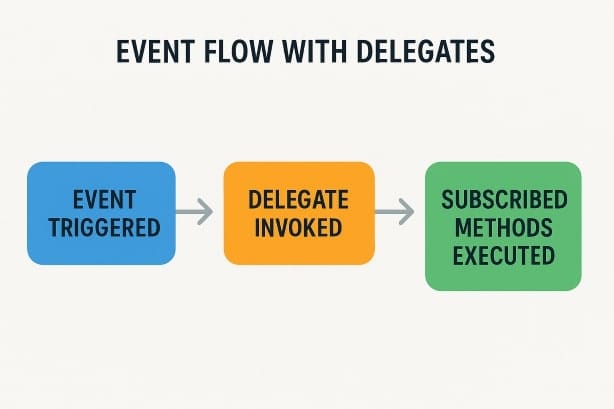
Practical Example: Implementing Events with Delegates
public class Process
{
public event Notify ProcessCompleted;
public void StartProcess()
{
Console.WriteLine("Process Started!");
// Process logic...
ProcessCompleted?.Invoke("Process Finished Successfully!");
}
}
Process process = new Process();
process.ProcessCompleted += ShowMessage;
process.StartProcess();
Explanation: When StartProcess
finishes, it notifies subscribers via the ProcessCompleted
event.
Common Pitfalls and Best Practices
- Null checking: Always check if a delegate is null before invoking (use
?.Invoke
). - Avoid exceptions in multicast delegates: One method throwing an exception can stop others.
- Keep delegates clean: Unsubscribe delegates to prevent memory leaks.
Advanced Delegate Concepts
You might think you’ve got delegates all figured out, but let me show you a few advanced tricks that can genuinely simplify your codebase and boost performance. Let’s dive deeper!
Generic Delegates (Func, Action, Predicate)
.NET gives you powerful generic delegates that cover 95% of your delegate needs — without cluttering your code with redundant definitions.
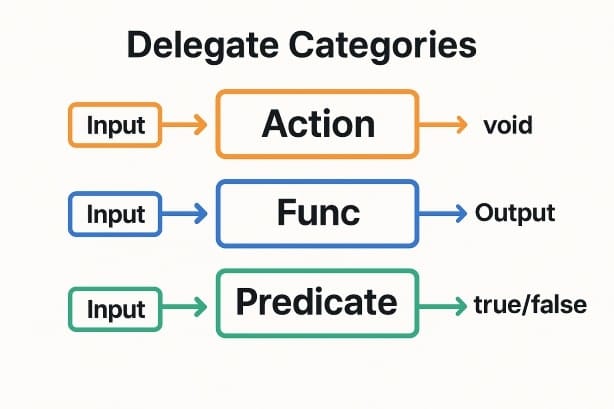
Here’s a quick breakdown:
- Action: Use this when you just want to do something, without caring about the return value.
- Func: This one’s perfect when you expect a result from your method.
- Predicate: Specifically checks a condition and returns a boolean — great for filters and validations.
Example in action:
// Action: Performs an action, returns nothing
Action<string> logMessage = message => Console.WriteLine(message);
logMessage("Hello from Action!");
// Func: Takes parameters, returns a value
Func<int, int, int> multiply = (a, b) => a * b;
int result = multiply(4, 5); // result = 20
// Predicate: Returns true/false
Predicate<int> isEven = number => number % 2 == 0;
bool check = isEven(10); // check = true
When to use:
- Replace verbose custom delegate types.
- Write cleaner and more reusable code.
- Improve readability, especially in LINQ and event handling scenarios.
Tip: These generic delegates are compiler-optimized. Less boilerplate, better performance!
Delegate Chaining
With chaining, you can invoke multiple methods sequentially through one delegate.
// Delegate declaration
delegate void Notify(string message);
// Single method
void ShowMessage(string message) => Console.WriteLine("Message: " + message);
// Chaining delegates
Notify notifier = ShowMessage;
notifier += msg => Console.WriteLine("Chained: " + msg);
// Invoke all methods in the chain
notifier("Delegate chaining in action!");
Use Cases:
- Event notifications — notify multiple subscribers.
- Logging systems — write to console, file, and external services simultaneously.
- Workflow sequences — execute a pipeline of operations.
Tip: Always handle exceptions inside chained methods. One failure can disrupt the entire chain!
Delegate Performance Considerations
Delegates in .NET are designed for speed, but there are a few performance best practices you shouldn’t ignore, especially at scale.
Optimize Delegate Usage:
- Reuse and cache delegates
Especially in loops, creating new delegate instances repeatedly can cause memory churn. - Minimize multicast chain length
Every method in the chain adds invocation overhead. Keep chains efficient. - Leverage generic delegates
Stick toAction
,Func
, andPredicate
where possible — they’re optimized and reduce clutter. - Benchmark critical paths
If your application heavily uses delegates (think: real-time processing, event systems), profile performance and optimize!
// Delegate caching example
Func<int, int> square = x => x * x;
for (int i = 0; i < 100000; i++)
{
int result = square(i); // Reusing delegate
}
Tip: Delegates are your performance friends, but always measure if they sit in hot paths of your application.
FAQ: Quick Answers to Common Delegate Questions
Absolutely! Delegates make excellent method parameters for flexible designs.
Action
returns void, Func
returns a value, Predicate
returns a bool.
Not by default. Use thread-safety mechanisms when modifying delegate invocation lists.
Yes! Use the -=
operator to unsubscribe.
Conclusion: Embrace Delegates for Cleaner, Flexible C# Code
As we’ve explored, delegates aren’t just an abstract concept — they’re a practical tool that powers real-world .NET applications. Whether you’re building event-driven systems or simply passing methods around for flexibility, mastering delegates will elevate your C# skills.
So here’s my challenge to you: go refactor a piece of your code using delegates and see how it improves readability and maintainability. And if you run into questions, drop a comment below — let’s discuss!