Installing Visual Studio
Installing Visual Studio has evolved over the past two decades. From the early days of Visual Studio .NET to the latest versions of Visual Studio 2022 and beyond, the installation process has become more streamlined and user-friendly.
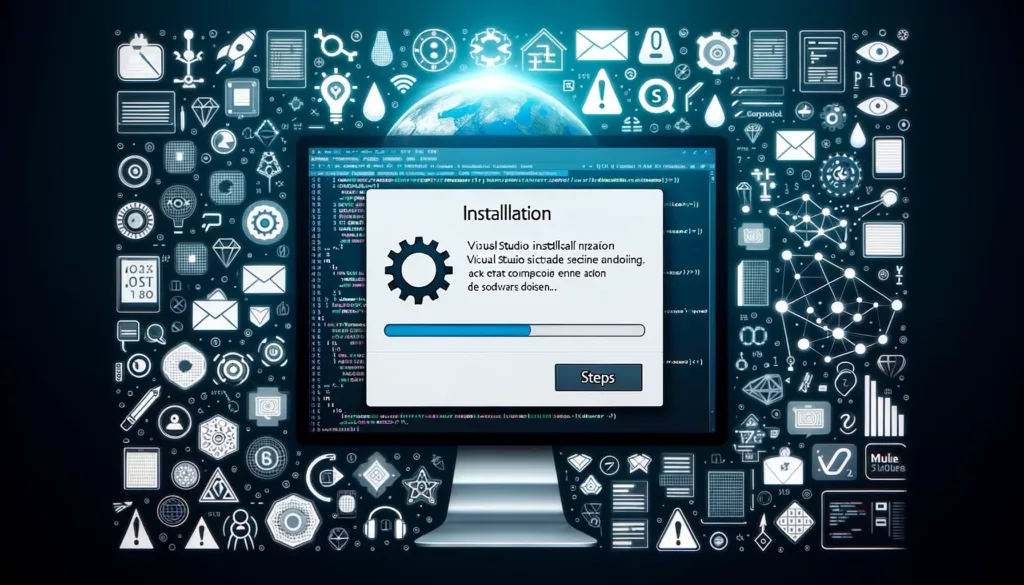
Here’s a more detailed guide to installing Visual Studio based on my experience:
- System Requirements:
- Before starting the installation, ensure that your system meets the recommended requirements for the Visual Studio version you’re installing.
- Download the Installer:
- Navigate to the official Visual Studio website.
- Decide on the edition you want:
- Visual Studio Community: Free edition for individual developers and students.
- Visual Studio Professional: Paid version with more features for professional developers.
- Visual Studio Enterprise: Comprehensive edition for large teams and enterprise solutions.
- Click on the “Download” button for your chosen edition.
- Run the Installer:
- Once downloaded, run the installer. Over the years, Microsoft has focused on making the installer smaller and faster. The latest versions have a bootstrapper that subsequently downloads necessary components.
- Choose Workloads:
- The installer will prompt you to select “workloads,” which are essentially predefined sets of tools and templates for specific types of development.
- For example:
- .NET desktop development for WPF, Windows Forms, and console applications.
- ASP.NET and web development for web applications, services, and APIs.
- Mobile development with .NET for Xamarin-based mobile apps.
- …and many more.
- Depending on your development goals, select the required workloads. You can always modify these selections later.
- Select Individual Components (optional):
- Beyond workloads, you can select individual components if you have specific needs. Most developers rely on the workloads, but this option is available for finer-grained control.
- Install Location:
- By default, Visual Studio will be installed on the C drive. If you need to change this, click on the “Options” button at the bottom to specify a different location. Make sure you have adequate disk space, especially if you’re installing multiple workloads.
- Start Installation:
- Click on the “Install” button. The installation might take some time, depending on your internet speed and the components you’ve selected.
- Reboot:
- In some cases, especially when certain system components or redistributables are updated, you might be prompted to reboot your computer.
- Launch Visual Studio and Initial Setup:
- After installation, start Visual Studio. On the first launch, you’ll be asked to sign in with a Microsoft account. While it’s optional for the Community edition, it provides synchronization of settings, themes, and access to additional resources for other editions.
- You’ll also be prompted to choose a development setting and theme. This determines the default layout and behavior of the IDE.
- Regular Updates:
- Over the years, I’ve noticed Microsoft is releasing updates more frequently. It’s crucial to keep Visual Studio updated not only for new features but also for performance improvements and security patches. Use the built-in update functionality in Visual Studio to check for and install updates.
Remember, installation is just the beginning. Over two decades, Visual Studio has grown into a powerful, versatile, and feature-rich IDE. It might seem overwhelming initially, but over time, its functionalities become indispensable for efficient development.
Creating Your First C# Project
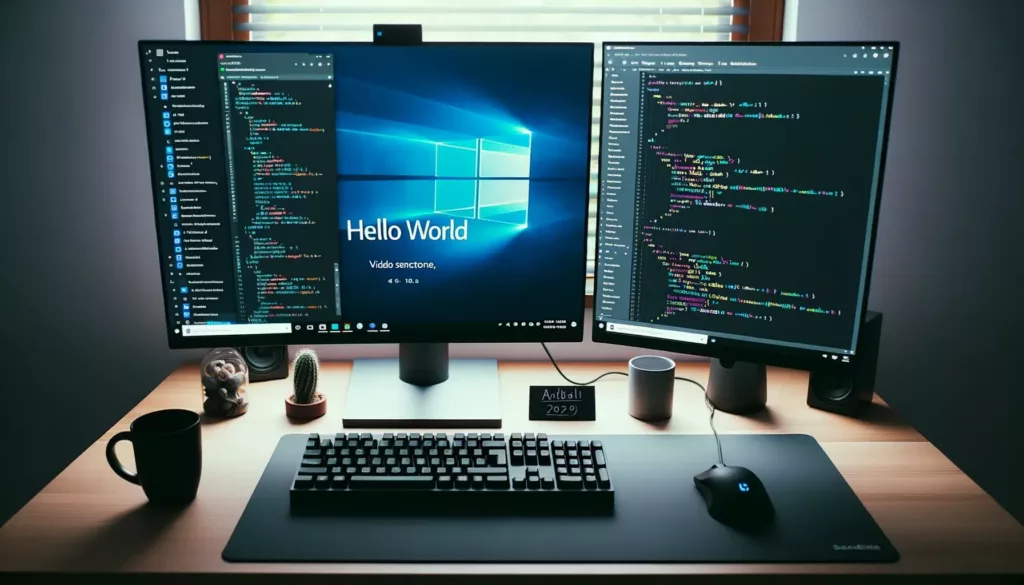
Let’s creating your first C# project in Visual Studio, along with some examples to get you started.
1. Launch Visual Studio
Once you have Visual Studio installed, launch it. You’ll be greeted with a welcome screen.
2. Create a New Project
- Click on the “Create a new project” option.
- In the search bar, type “C#”.
- From the list of templates, choose “Console App (.NET Core)” for a cross-platform console application or “Console App (.NET Framework)” if you are specifically targeting Windows.
- Click on the “Next” button.
3. Configure Your Project
- Give your project a name, e.g., “HelloWorld”.
- Choose a location on your computer where you’d like the project files to be saved.
- Click on the “Create” button.
4. Writing Your First C# Code
Visual Studio will now generate a new console project for you. You should see a file named Program.cs
open in the editor, containing code similar to:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
This program will simply print “Hello World!” to the console when run.
5. Understanding the Code
Here’s a brief overview of what the generated code means:
- using System;: This imports the
System
namespace, giving you access to various fundamental classes, including theConsole
class. - namespace HelloWorld: This declares a new namespace named
HelloWorld
. Namespaces are used to organize code and prevent naming collisions. - class Program: This declares a new class named
Program
. Classes are fundamental building blocks in C# and encapsulate data for the object and methods to manipulate that data. - static void Main(string[] args): This is the Main method, the entry point of the application. When you run your program, it starts executing from here.
- Console.WriteLine(“Hello World!”);: This writes the string “Hello World!” to the console window.
6. Run Your Program
- Press
F5
or click the green play button at the top. This will build and run your program. - A console window should appear displaying “Hello World!”.
- Press any key to close the console window.
7. Modify the Program
Let’s make a small modification. We’ll prompt the user for their name and greet them:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("What is your name?");
var name = Console.ReadLine();
Console.WriteLine($"Hello, {name}!");
}
}
}
Now, when you run the program, it will ask for your name and greet you personally.
Final Thoughts
The journey of becoming proficient in C# and .NET is continuous, but it’s also rewarding. The platform’s versatility and robustness, combined with the powerful tools provided by Visual Studio, make it one of the top choices for developers around the world. Keep learning and building!
Exploring the Visual Studio IDE
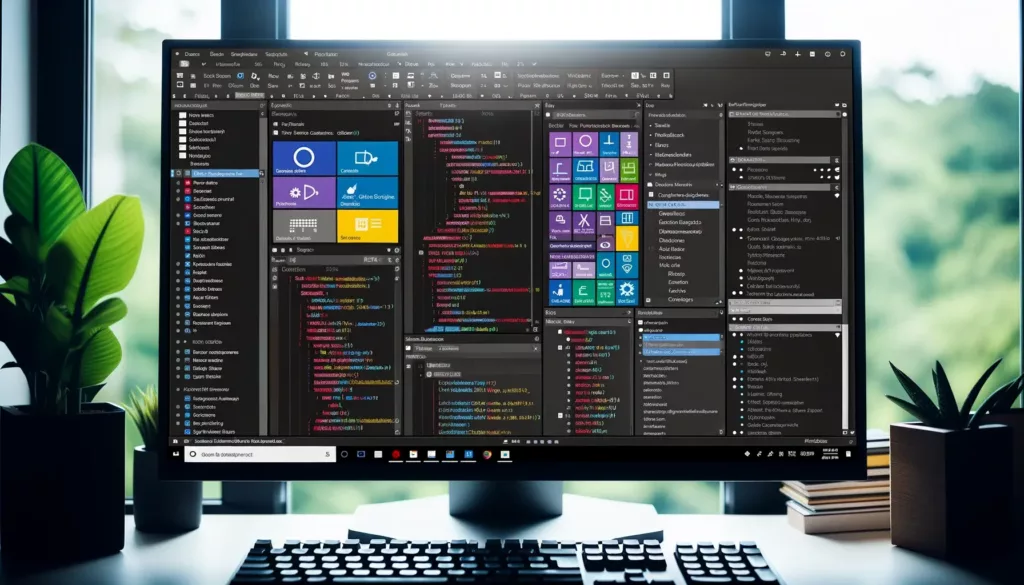
More that 20 years Visual Studio grow from its early stages to the powerful integrated development environment (IDE) that it is today. Here’s a comprehensive overview of the Visual Studio IDE, emphasizing the most vital areas you should be familiar with:
1. Solution Explorer:
- This is your primary navigation tool. Here, you’ll see a hierarchical view of your solution, projects, references, and individual files.
- Right-clicking on items provides context-specific options, like adding references, new items, or even renaming.
2. Code Editor:
- Where you’ll spend the majority of your time writing and reading code.
- Supports IntelliSense, which provides code suggestions, definitions, and auto-completion based on context.
- Supports split view, where you can view two sections of a file (or two files) side by side.
3. Properties Window:
- Displays properties of selected items (like controls in a Windows Forms project).
- Allows for interactive editing of these properties.
4. Toolbox:
- Provides drag-and-drop controls/components for design surfaces (like Windows Forms or WPF).
- It’s context-sensitive, showing controls relevant to the current editor.
5. Server Explorer:
- Connects to databases, servers, and other external services. Useful for database-centric applications.
6. Output Window:
- Displays output from build processes, debug information, and other messages.
- Very helpful for troubleshooting build or deployment issues.
7. Error List Window:
- Lists all errors, warnings, and messages. Clicking on an item will take you directly to the offending code line.
8. NuGet Package Manager:
- Manage external libraries and tools in your projects.
- Can be accessed via
Tools
>NuGet Package Manager
or right-clicking the solution/project in Solution Explorer.
9. Debugging Tools:
- The toolbar at the top provides debug controls like Start, Stop, Pause, Step Over, and Step Into.
- Breakpoints: Clicking to the left of a code line in the editor sets a breakpoint. Execution pauses when it hits this line.
- Immediate Window: Allows for real-time evaluation of code while paused in debug mode.
- Locals and Watch Windows: Show variable values and allow you to monitor specific variables, respectively.
- Call Stack: Shows the active stack trace during debugging, allowing you to navigate through the chain of method calls.
10. Extensions and Updates:
- Visual Studio has a vast marketplace of extensions that can enhance or modify its capabilities.
- Access via
Extensions
>Manage Extensions
. - It’s essential to keep extensions updated, as they can affect IDE performance.
11. Team Explorer:
- If you’re using version control (like Git), this window provides an interface to interact with repositories, view changes, history, branches, and more.
- Integrates with Azure DevOps (previously Visual Studio Team Services and Team Foundation Server).
12. CodeLens:
- Above methods or classes, you’ll sometimes see references, changes, or other annotations. This feature provides insights directly in the code editor.
- Particularly useful in team settings to understand who modified code and why.
13. Refactoring Tools:
- Visual Studio offers powerful tools to refactor code, like renaming variables/methods/classes, extracting methods, and implementing interfaces.
14. Editor Configurations and Shortcuts:
- Over the years, I’ve found customizing the editor (font, size, color schemes) beneficial. This can be done under
Tools
>Options
. - Familiarity with shortcuts (like
Ctrl+Space
for IntelliSense orCtrl+K, Ctrl+C
to comment code) drastically boosts productivity.
15. Live Share:
- A relatively newer feature that lets you collaborate on code in real-time with other developers, much like Google Docs but for code.
Closing Thoughts:
Visual Studio is not just an editor; it’s a complete environment to streamline the development process. The more you delve into its features and tools, the more productive you will become. My advice to newcomers: Spend time understanding the IDE. A well-understood tool is a powerful ally in software development.
FAQ
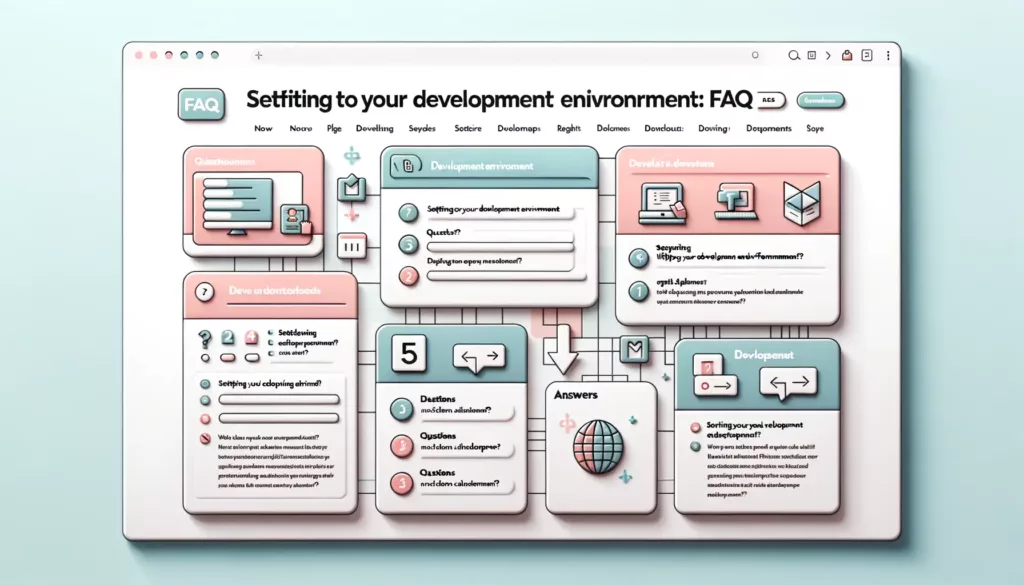
A development environment refers to the collection of software and configurations on a computer used to develop software applications. It typically includes an integrated development environment (IDE), a compiler or interpreter for the chosen language, and any necessary dependencies or libraries.
A properly set up environment ensures that you can focus on coding without interruptions, reduces bugs related to environmental discrepancies, and generally provides a smoother and more productive coding experience.
Common components include:
1. An IDE or code editor (e.g., Visual Studio, Eclipse, VS Code).
2. A version control system (e.g., Git).
3. Compilers or interpreters for your language of choice.
4. Libraries or frameworks required for your project.
5. Development server (for web development).
6. Database management systems (if applicable).
Your choice should be based on the programming language you’re using, the platform you’re targeting, and your personal preferences. Some popular IDEs include Visual Studio (C#), IntelliJ IDEA (Java), and PyCharm (Python).
Version control systems track changes in your codebase, allowing multiple developers to work on the same project without conflicts and enabling you to revert to previous versions of your code. Git is a widely-used version control system.
For many web applications, yes. A local server allows you to test and develop your site/app without the need to deploy to a live server. Tools like Apache, Nginx, or built-in development servers in frameworks can be used.
Databases can be local or remote. Tools like MySQL, PostgreSQL, and SQLite can be installed locally. Cloud providers also offer managed database solutions. Always ensure you have proper backups and use seed/migration scripts for consistency.
Ideally, yes. This reduces the chances of code working in development but failing in production due to environmental differences. Tools like Docker can help mirror production environments locally.
1. Always check error messages thoroughly.
2. Ensure all services and servers are running.
3. Check for updates or changes in libraries or tools you’re using.
4. Consider seeking help online, such as on Stack Overflow, but ensure sensitive information remains confidential.
Regularly check for updates for your tools and libraries. However, before updating, ensure compatibility and check if there are any breaking changes.
Most modern tools and languages support Windows, macOS, and Linux. However, some tools might be OS-specific, so always check compatibility.