Have you ever launched your .NET MAUI app and wondered what actually happens behind the scenes? Spoiler: It’s way more than just showing the first page. Whether you’re a newcomer or already have a few MAUI apps in production, mastering the app lifecycle is crucial to build responsive and efficient applications. Let’s uncover the secrets!
What is App Lifecycle in .NET MAUI?
The app lifecycle refers to the stages an application goes through from start to finish. Think of it like the lifecycle of a butterfly: egg, larva, pupa, and finally adult butterfly (beautiful app!). Understanding these stages ensures that your app behaves predictably across different devices and platforms.
Definition and Core Concepts
- Lifecycle is the process your app follows from launch to termination.
- It includes events like startup, running, backgrounding, resuming, and shutdown.
- Proper handling of these events ensures good user experience and resource management.
- Lifecycle management helps you tackle real-world problems like app crashes, data loss, and performance bottlenecks.
Comparison to Other Frameworks
- Xamarin.Forms: MAUI builds upon Xamarin.Forms but provides a more unified lifecycle experience with fewer platform-specific quirks.
- WPF: WPF apps typically run on desktops where lifecycle is simpler, but MAUI needs to handle mobile app suspensions and terminations more gracefully.
- UWP: Similar to UWP, MAUI apps must manage state carefully, as they can be terminated at any moment by the OS.
Lifecycle Events in .NET MAUI
Application Startup: What Happens First?
CreateMauiApp()
sets up the entire application environment.- Services, dependencies, fonts, and configurations are initialized here.
- Logging and error tracking services can also be configured at this stage.
Initialization: Setting the Scene
- The constructor of
App.xaml.cs
is triggered. - Here, you set up navigation containers, dependency injection, and essential services.
- Example: Initializing analytics tracking or loading cached user settings.
App Resumed: Waking Up the App
OnResume()
is called when the user returns to the app.- Great moment to check if data is stale and needs refreshing.
- Use this to restart animations or re-establish lost connections.
App Sleep: Preparing for Pause
OnSleep()
runs when the app is about to enter the background.- Ideal for saving unsaved user data, pausing media playback, and releasing memory-heavy objects.
Stop and Shutdown: The Graceful Exit
- While shutdown events are not guaranteed,
OnSleep()
acts as your safety net. - If supported by the platform, ensure logs are flushed and critical operations are completed.
Deep Dive: .NET MAUI Lifecycle Methods
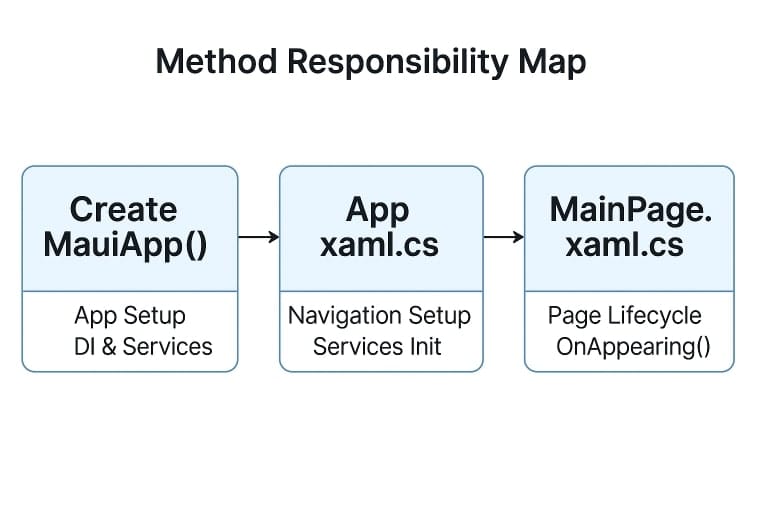
Understanding CreateMauiApp()
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
})
.ConfigureServices(services =>
{
services.AddSingleton<IMyService, MyService>();
});
return builder.Build();
}
Explanation: This is where your app gets its foundation. Services, fonts, logging — everything begins here. This ensures consistency across all platforms.
Role of App.xaml.cs
in Lifecycle
public App(IMyService myService)
{
InitializeComponent();
MainPage = new NavigationPage(new MainPage(myService));
}
Explanation: Sets up navigation and defines the root page of your app. Ideal place to pass injected services to your pages.
Using OnStart()
, OnSleep()
, OnResume()
protected override void OnStart() {
// Analytics tracking or initial data load
}
protected override void OnSleep() {
// Save app state and release non-critical resources
}
protected override void OnResume() {
// Refresh data and reconnect to services
}
Explanation: Lifecycle hooks let you respond to changes in app state with precision.
Handling Lifecycle in MainPage.xaml.cs
protected override void OnAppearing()
{
base.OnAppearing();
// Update UI with latest data, start animations
}
Explanation: This ensures your page feels alive and responsive every time it’s presented.
Dependency Injection & Lifecycle
Scoped vs Singleton Services
- Singleton: Created once and shared across the entire app.
- Scoped: Created once per request or scope, perfect for per-page services.
Injecting Services in App.xaml.cs
public App(IMyService myService)
{
InitializeComponent();
MainPage = new MainPage(myService);
}
Explanation: Dependency injection promotes cleaner architecture and testability.
Extra Tip: Use Transient
services when you need fresh instances for short-lived operations.
State Management Across Lifecycle
Saving and Restoring State
- Preferences: Store non-sensitive app settings.
- Secure Storage: Store sensitive data like tokens securely.
Preferences.Set("theme", "dark");
var theme = Preferences.Get("theme", "light");
Handling Transient State
- Use in-memory caches or temporary files for transient states.
- Consider using MVVM frameworks like CommunityToolkit.MVVM to bind and manage state seamlessly.
Tip: Serialize complex objects into JSON and store them in Preferences.
Lifecycle Event Handling Best Practices
- Minimize startup time: Avoid synchronous heavy operations. Prefer async loading.
- Manage resources efficiently: Dispose of timers, background services, and database connections in
OnSleep()
. - Dealing with background tasks: Use
IBackgroundTask
libraries to run safe, background processes. - Test on multiple devices: Simulate backgrounding and resuming on both Android and iOS for best results.
Common Pitfalls and How to Avoid Them
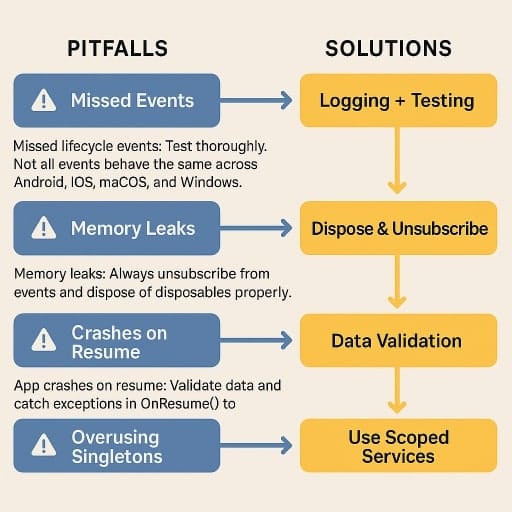
- Missed lifecycle events: Test thoroughly. Not all events behave the same across Android, iOS, macOS, and Windows.
- Memory leaks: Always unsubscribe from events and dispose of disposables properly.
- App crashes on resume: Validate data and catch exceptions in
OnResume()
to prevent crashes. - Overusing Singleton: Avoid singletons for per-page services to prevent state pollution.
Real-World Example: Building a Responsive MAUI App
Imagine building a note-taking app:
- Startup: Load cached notes and prepare the UI.
- OnResume: Refresh notes from the cloud and check connectivity.
- OnSleep: Save unsaved notes to local storage and release background resources.
protected override void OnStart() {
notesService.LoadCachedNotes();
}
protected override void OnResume() {
notesService.RefreshNotesFromCloud();
}
protected override void OnSleep() {
notesService.SaveNotesLocally();
notesService.CleanupResources();
}
Explanation: A robust approach to ensure your data stays fresh and safe, even when the app state changes.
FAQ: App Lifecycle in .NET MAUI
OnStart()
to initialize everything?Not recommended. Keep heavy lifting for CreateMauiApp()
or asynchronous background tasks.
Initialize push notification listeners in OnResume()
to catch notifications while app is active.
Absolutely! Use platform-specific services or Essentials library for background processing.
Add comprehensive logging in each lifecycle method to trace app behavior across transitions.
Yes! Serialize to JSON and store in Preferences or local database like SQLite.
Conclusion: Mastering .NET MAUI Lifecycle
Understanding the .NET MAUI app lifecycle isn’t just theory — it’s your roadmap to building smooth, reliable applications. Start using these techniques today to make your apps snappy and robust!
What has been your biggest challenge with the MAUI lifecycle so far? Drop a comment below — let’s discuss!