Do you think arrays are just basic containers in C#? Think again! They are powerful tools that, when used correctly, can make your code cleaner, faster, and far more elegant. Today, I’m going to show you how to unleash the true power of arrays in C#. Let’s dive in!
Introduction to C# Arrays
Arrays in C# aren’t just simple containers — they’re high-speed, memory-friendly structures that give you direct control over your data. Imagine having a toolbox where every tool is purpose-built for lightning-fast access and manipulation. That’s what arrays bring to the table.
Technically, an array is a fixed-size collection of elements, all of the same type, stored in a contiguous block of memory. This physical memory alignment is what makes array operations blazingly fast compared to many other collection types.
Key Benefits:
- Constant-time access (
O(1)
) to any element by its index. - Lower memory overhead since elements are tightly packed.
- Compatibility with low-level operations and interop scenarios.
And here’s a bonus from my experience: Arrays are incredibly cache-friendly. In large-scale data processing tasks, this can reduce CPU cache misses and dramatically speed up your application.
Fun fact: Under the hood, even advanced structures like
List<T>
use arrays for storage. So, understanding arrays is foundational for mastering .NET collections as a whole.
Basic Concepts of Arrays in C#
An array is like a row of mailboxes. Each mailbox (element) has an address (index). In C#, arrays are zero-indexed, meaning the first element is at index 0
.
int[] numbers = new int[3];
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
Explanation:
- We declare an array of integers with a size of 3.
- Each index is assigned a value individually.
Arrays can store any data type, including user-defined objects, making them versatile.
string[] names = { "Alice", "Bob", "Charlie" };
Explanation: Using array initializers simplifies code and improves readability.
Important Note: Arrays are reference types in C#. When you pass an array to a method, you’re passing a reference, not a copy. Changes inside the method affect the original array.
Creating and Initializing Arrays
There are multiple ways to create and initialize arrays in C#:
- Static Initialization: When you know the values upfront.
int[] ages = new int[] { 25, 30, 35 };
- Simplified Syntax: Even cleaner when types can be inferred.
int[] ages = { 25, 30, 35 };
- Dynamic Initialization: Useful when the values come from runtime computations or user input.
int size = 5;
int[] dynamicArray = new int[size];
for (int i = 0; i < size; i++)
{
dynamicArray[i] = i * 10;
}
Tip from experience: Dynamic initialization is fantastic when you work with user inputs or data from external sources. In many real-world systems, like processing sensor arrays or parsing dynamic data sets, this becomes invaluable.
Arrays can even be initialized using loops or LINQ expressions, giving you flexibility during runtime operations.
Multidimensional and Jagged Arrays
When data is tabular or hierarchical, you’ll want more than a single-dimension array.
Multidimensional Array
int[,] matrix = new int[2, 3] { { 1, 2, 3 }, { 4, 5, 6 } };
Explanation:
- Think of this as a grid with 2 rows and 3 columns.
- Useful for mathematical computations, like matrix operations, image processing, or game maps.
Jagged Array (Array of Arrays)
int[][] jaggedArray = new int[2][];
jaggedArray[0] = new int[] { 1, 2 };
jaggedArray[1] = new int[] { 3, 4, 5 };
Explanation:
- Each row can have a different number of elements.
- Perfect for scenarios like handling hierarchical data, where child arrays can vary in length (e.g., student scores per class).
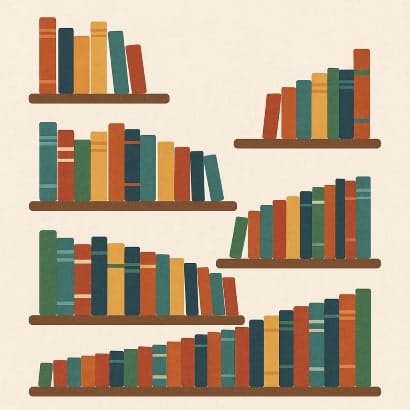
Note: In performance-sensitive applications, jagged arrays are often preferred over multidimensional arrays because they can be slightly faster and more memory-efficient.
Common Operations on Arrays
Working with arrays becomes powerful when you leverage built-in methods and techniques.
- Iteration: Looping through elements.
foreach (var name in names)
{
Console.WriteLine(name);
}
- Sorting: Organize your array for quick searches.
Array.Sort(ages);
- Searching: Find the index of an element.
int index = Array.IndexOf(ages, 30);
- Reversing: Reverse the order of elements.
Array.Reverse(ages);
- Copying: Clone an array for safe modifications.
int[] copy = new int[ages.Length];
Array.Copy(ages, copy, ages.Length);
Tip: Before implementing your own algorithms, check
System.Array
methods. They cover most standard operations, and they are highly optimized.
Advanced Topics
Ready to go beyond the basics? Here are some advanced patterns:
Using LINQ with Arrays
var filtered = ages.Where(age => age > 25).ToArray();
Explanation: LINQ (Language Integrated Query) provides readable, declarative code for data querying and transformations.
Parallel Processing
Parallel.For(0, ages.Length, i =>
{
ages[i] *= 2;
});
Explanation: Useful in CPU-intensive operations, this speeds up processing by distributing work across multiple cores.
Span for Performance
Span<int> span = ages;
span[0] = 99;
Explanation: Span<T>
enables slicing and high-performance memory access without extra allocations. Ideal for performance-critical applications like parsers or streaming data processors.
Bonus: Explore
Memory<T>
for scenarios involving asynchronous data processing.
Real-world Applications and Best Practices
Arrays shine in many production environments:
- Game Development: Arrays are frequently used for storing game states, player data, or level designs.
- Data Analysis Tools: Arrays handle large datasets efficiently, especially for numerical computations.
- Embedded Systems: Limited resources? Arrays provide deterministic memory usage.
- Streaming and Buffering: Audio/video streams often use circular arrays as buffers.
Best Practices:
- Prefer arrays when you know the size and need optimal performance.
- Combine arrays with LINQ or
Span<T>
for readable yet performant code. - Regularly validate indices to prevent runtime errors.
From my experience in optimizing data-heavy applications, replacing dynamic lists with fixed-size arrays in critical paths cut processing time by nearly half!
FAQ: Common Questions About C# Arrays
Arrays are fixed in size. You can use Array.Resize()
, which creates a new array under the hood. Alternatively, for dynamic scenarios, consider using List<T>
.
Numeric types default to 0, booleans to false
, and reference types to null
. It’s crucial to initialize arrays explicitly when needed.
Always check array.Length
before accessing an index, or use safer constructs like TryGetValue
with collections.
Use arrays for predictable, fixed-size collections with performance focus. Use List<T>
for dynamic, resizable collections.
No, by default they are not. Use synchronization mechanisms or concurrent collections for multi-threaded access.
Conclusion: Mastering C# Arrays for Clean, Efficient Code
As you’ve seen, arrays in C# are far more than just basic data containers. They’re tools of precision, performance, and practicality. Whether you’re building a high-performance game engine, processing massive datasets, or optimizing for low-level operations, arrays will be your trusted companion.
Next time you reach for an array, think beyond just storing data. Use the tips, tricks, and best practices from this guide to write cleaner and faster code.
Have you faced interesting challenges with arrays in your projects? Share your stories in the comments! Let’s learn together.