Have you ever thought you’re mapping your .NET database models the “right” way — only to find hidden issues months later? If you’re relying purely on Fluent API or letting EF Core “figure it out” by convention, you’re probably missing a trick. Today, we’re diving deep into the world of Entity Framework Data Annotations — your shortcut to smarter, cleaner, and more maintainable database mapping!
Understanding Entity Framework Configuration: Data Annotations vs Fluent API
Entity Framework (EF) Core offers two primary ways to configure your models: Data Annotations and Fluent API. Each approach has distinct strengths and ideal use cases, and understanding the nuances between them can dramatically improve the quality, maintainability, and scalability of your application.
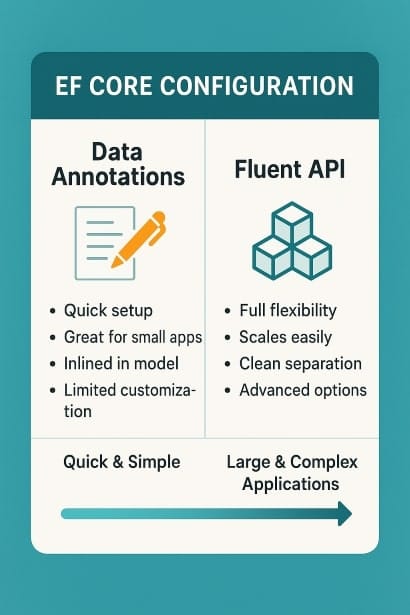
While Data Annotations are perfect for quick and straightforward configurations embedded directly into your domain models, Fluent API provides a far more detailed and versatile way to define relationships, constraints, and behaviors externally. Mastering both techniques ensures that you can choose the right strategy for any scenario, whether it’s a lightweight proof-of-concept or a full-scale enterprise solution.
By strategically selecting between Data Annotations and Fluent API based on project complexity and future needs, you’ll set yourself up for smoother development cycles, fewer bugs, and a more robust architecture overall. Let’s explore them in depth and find out when and how to use each one to your advantage.
What Are Data Annotations?
Data Annotations are attributes you add directly to your C# classes and properties. They’re straightforward, simple, and perfect for quick configurations.
Example: Defining a Required Property and a Maximum Length
public class Product
{
public int Id { get; set; }
[Required]
[MaxLength(100)]
public string Name { get; set; }
}
Explanation:
[Required]
ensures theName
field cannot be null.[MaxLength(100)]
limits theName
to 100 characters.
When to use:
- Quick validations.
- Small projects.
- When you want to keep configuration tightly coupled with the model.
Data Annotations in Depth
Data Annotations offer a variety of options to define rules and constraints right within your model classes. Here’s a breakdown of commonly used annotations:
- [Required] — Makes a property mandatory.
- [MaxLength]/[MinLength] — Restricts string length.
- [StringLength] — Combines minimum and maximum length.
- [Range] — Sets a numerical range constraint.
- [Key] — Marks a property as the primary key.
- [ForeignKey] — Specifies foreign key relationships.
- [Column] — Maps the property to a specific column name and order.
- [Table] — Maps a class to a specific table name.
Tip: Use annotations for straightforward rules, but avoid cluttering your model with complex configuration logic.
Fluent API: The Heavyweight Champion
The Fluent API gives you full control over model configuration — all while keeping your domain models clean.
Example: Configuring via Fluent API
public class AppDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>(entity =>
{
entity.Property(e => e.Name)
.IsRequired()
.HasMaxLength(100);
});
}
}
Explanation:
.IsRequired()
ensures theName
cannot be null..HasMaxLength(100)
limits the character length.
When to use:
- Larger, more complex projects.
- Keeping entity classes clean.
- When configurations depend on external factors or conventions.
Fluent API in Depth
The Fluent API allows you to build highly customized and expressive configurations using method chaining. Key configurations include:
- Property Configuration: Control nullability, length, types, default values.
- Relationship Configuration: Define one-to-one, one-to-many, many-to-many relations.
- Keys and Indexes: Set up composite keys, unique constraints, and indexes.
- Table and Column Mapping: Specify custom table names, schemas, and column configurations.
- Shadow Properties: Configure properties not defined in the model class.
- Global Conventions: Apply rules across multiple entities programmatically.
Tip: Organize Fluent API configurations into separate classes implementing
IEntityTypeConfiguration<TEntity>
for clean architecture and better maintainability.
When to Use Data Annotations vs Fluent API
Choosing between Data Annotations and Fluent API depends on the project’s size, complexity, and maintainability goals.
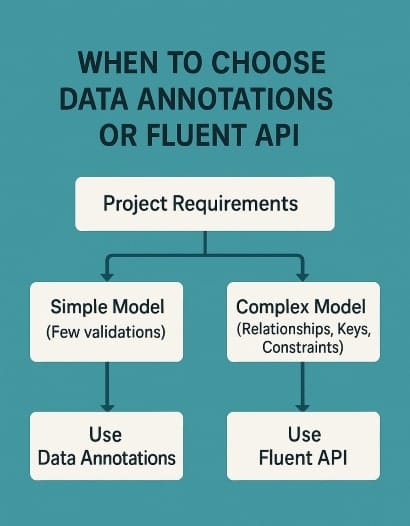
Use Data Annotations when:
- Your model is simple and needs minor validations.
- You want faster setup for small applications.
- Minimal configuration is required, making attributes sufficient.
Use Fluent API when:
- The model relationships are complex (multiple keys, inheritance, shadow properties).
- You need separation of concerns (keeping entities clean).
- You require advanced configurations (like computed columns, alternate keys, etc.).
Example: Data Annotations vs Fluent API
Data Annotations:
public class Customer
{
[Key]
public int CustomerId { get; set; }
[Required, MaxLength(50)]
public string Email { get; set; }
}
Fluent API:
modelBuilder.Entity<Customer>(entity =>
{
entity.HasKey(e => e.CustomerId);
entity.Property(e => e.Email)
.IsRequired()
.HasMaxLength(50);
});
Both achieve the same outcome, but Fluent API offers more flexibility if future changes are anticipated.
Pros and Cons: Data Annotations vs Fluent API
Feature | Data Annotations | Fluent API |
---|---|---|
Ease of Use | Simple and quick | More verbose |
Control | Limited | Full control |
Separation of Concerns | Mixed into model | Clean separation |
Scalability | Limited for complex scenarios | Excellent |
Common Pitfalls and Best Practices
Mixing Both Approaches Indiscriminately
While you can combine Data Annotations and Fluent API, avoid configuring the same property in both ways.
Bad Practice:
public class Product
{
[Required]
public string Name { get; set; }
}
// Fluent API overrides:
modelBuilder.Entity<Product>()
.Property(p => p.Name)
.HasMaxLength(200);
Explanation: This causes confusion because different configurations might lead to unpredictable behavior.
Best Practice: Choose one method per property.
Over-annotating Entities
Adding too many attributes clutters your domain models.
Bad Practice:
public class Customer
{
[Required]
[MaxLength(50)]
[StringLength(50)]
[Column("CustomerEmail")]
public string Email { get; set; }
}
Best Practice: Move complex configuration into Fluent API.
modelBuilder.Entity<Customer>(entity =>
{
entity.Property(e => e.Email)
.IsRequired()
.HasMaxLength(50)
.HasColumnName("CustomerEmail");
});
Forgetting Indexes
Indexes are vital for query performance but often overlooked.
Example:
modelBuilder.Entity<Product>()
.HasIndex(p => p.Name)
.IsUnique();
Explanation: Without an index, frequent queries on Name
would result in slower lookups.
Managing Table Names and Schema
Don’t leave EF’s default pluralization unless it matches your DB naming conventions.
Example:
modelBuilder.Entity<Product>()
.ToTable("Product", "inventory");
Explanation: Mapping explicitly ensures clean and consistent database structure, especially when working across teams.
Real-World Scenario: Choosing the Right Tool
When I was building an inventory management system, the initial models were simple — a few [Required]
and [MaxLength]
attributes were enough.
As complexity grew — multiple keys, indexes, shadow properties — Fluent API became essential. Separating configuration into EntityTypeConfiguration
classes not only kept things organized but made future updates painless.
Example: Early simple model with Data Annotations
public class InventoryItem
{
public int Id { get; set; }
[Required]
[MaxLength(200)]
public string ItemName { get; set; }
[Required]
public int Quantity { get; set; }
}
As the project grew, the Fluent API configuration evolved:
public class InventoryItemConfiguration : IEntityTypeConfiguration<InventoryItem>
{
public void Configure(EntityTypeBuilder<InventoryItem> builder)
{
builder.ToTable("InventoryItems", "warehouse");
builder.HasKey(x => x.Id);
builder.Property(x => x.ItemName)
.IsRequired()
.HasMaxLength(200);
builder.Property(x => x.Quantity)
.IsRequired();
builder.HasIndex(x => x.ItemName)
.IsUnique();
}
}
Explanation:
- Clean separation of configuration logic from the entity class.
- Defined indexes and constraints more explicitly.
- Made it easier to modify and extend without touching the entity model.
Lesson learned: Start simple but be ready to migrate to Fluent API as your project scales.
FAQ: Quick Answers About EF Configuration
Yes, but be careful not to configure the same property twice.
Performance is the same — it’s all about maintainability.
In enterprise applications or when you need precise control.
Absolutely. Fluent API takes precedence during runtime.
Highly recommended for large projects! It keeps your DbContext
clean and scalable.
Conclusion: Master Configuration and Build Better Applications
Understanding and properly using Data Annotations and Fluent API will not only make your Entity Framework Core models cleaner but will also prevent many future headaches.
Start smart, scale smarter! I challenge you to review your current models: are they using the best configuration method for their complexity?
Let’s discuss below — do you prefer Data Annotations or Fluent API in your projects?
- Introduction to EF Core: Power Up Your .NET Data Access
- Install EF Core Fast: Step-by-Step Beginner’s Guide
- Entity Framework Data Annotations & Fluent API Configuration
- EF Core Global Filters: Soft Delete & Multi‑Tenant Guide
- EF Core Transactions Guide & Code Examples (2025)