Introduction to Object-Oriented Programming (OOP) in C#
Object-Oriented Programming (OOP) in C# is a programming paradigm centered around objects rather than actions. The idea is to bundle data and the methods that operate on that data into one place. Imagine you are a chef with a recipe box. Each recipe card contains not only the list of ingredients (data) but also the instructions (methods) for making the dish. In C#, these recipe cards are akin to classes, and the dishes you make with them are the objects. Encapsulation, Inheritance and Polymorphism are three fundamental concepts of OOP.
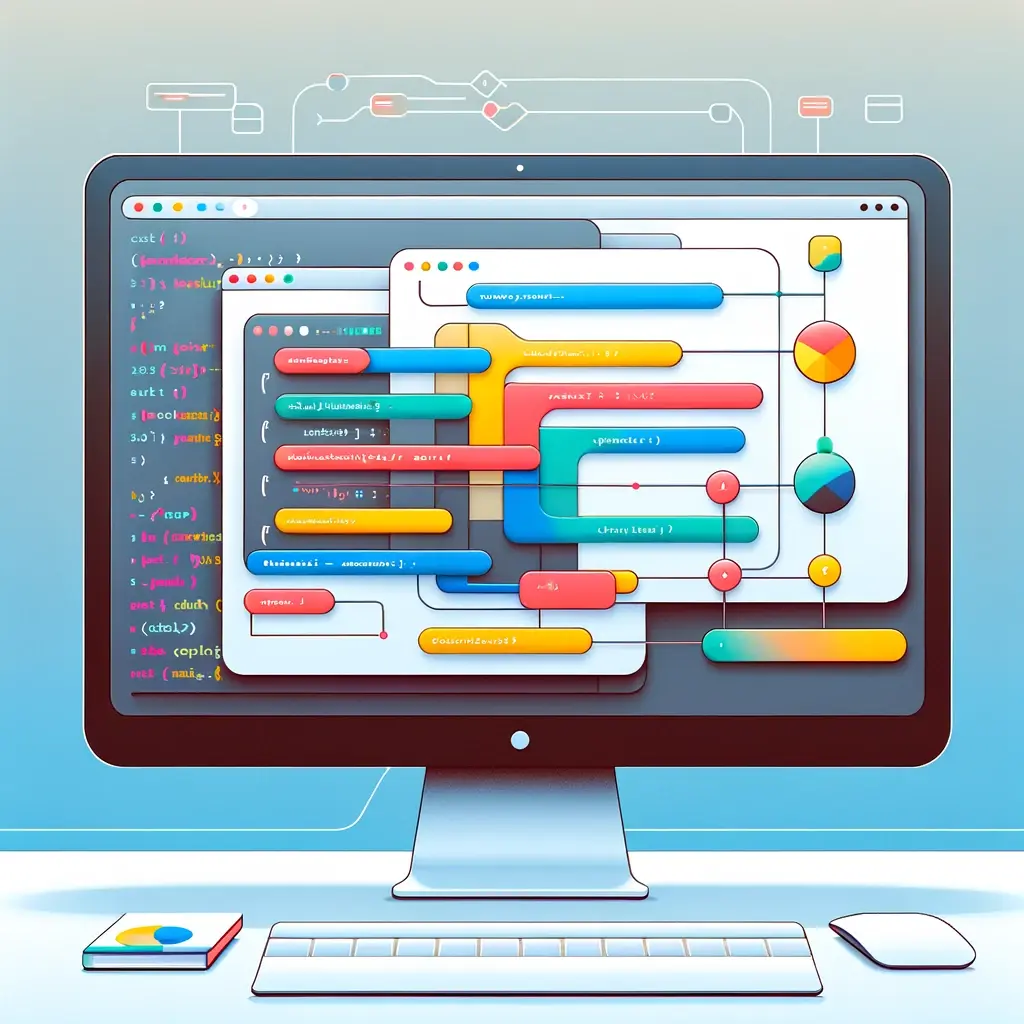
Encapsulation
In C#, encapsulation fundamentally ensures that a class hides its internal workings from the outside. Think of it like packing a surprise gift; you know you’ll get something special, but you don’t see the contents until you actually open it. This concept has several clear benefits:
- Simplicity: When using a class, you don’t need to understand the complexity inside. Just like using a smartphone, you don’t need to know how it works internally to send a message. Encapsulation allows users to operate with the confidence that the class will behave in the expected way.
- Protection: It keeps the class’s internal data safe from unwanted interference. Just as you wouldn’t arbitrarily change someone’s saved game files, encapsulation ensures that you can’t tamper with a class’s internal state unexpectedly, maintaining data integrity.
- Flexibility and Maintenance: It allows the developer to change internal workings without worrying about it affecting other parts of the program. Much like you can update your kitchen appliances without remodeling the entire kitchen, you can update and improve a class internally without altering its external interface.
These benefits make encapsulation a vital part of writing robust, user-friendly C# applications.
For example, think of a class as a wrapped box (class Circle
). You can’t see inside the box (private
variables) directly, but you can use a set of provided functions (like GetRadius()
) to interact with its contents.
public class Circle
{
private double radius;
public double GetRadius()
{
return radius;
}
public void SetRadius(double value)
{
if (value >= 0)
radius = value;
}
}
In the example above, the actual radius
field is hidden and access is provided through the GetRadius()
and SetRadius()
methods, ensuring that negative values cannot be set.
Inheritance
Inheritance in C# is a fundamental concept of object-oriented programming that allows a new class, known as a derived class, to inherit the members (fields, properties, methods, events) of an existing class, referred to as the base class. This mechanism provides a way to create a new class from an existing class but with additional or modified functionality.
Here are three specific benefits of using inheritance in C#:
- Code Reusability: Inheritance supports the reuse of code across classes. Instead of writing the same code over and over for each new class, you can create a common base class with shared code and then inherit that base class wherever needed. For example, you could have a base class
Animal
that has methods such asEat()
andSleep()
, and then have a derived classBird
that inherits these methods and addsFly()
. - Simplified Maintenance: When common functionality is located in the base class, maintaining and updating the code becomes easier. Any change you make to the base class automatically propagates to the derived classes, reducing the potential for error when making changes. If a method in the base class
Vehicle
is updated, all derived classes likeCar
andTruck
automatically get the updated behavior. - Polymorphism: Inheritance enables polymorphism, where a single operation can behave differently on different classes. For instance, a base class
Shape
might have a methodDraw()
, and its derived classes likeCircle
,Square
, andTriangle
can implement their own versions ofDraw()
. This allows the same call toDraw()
to produce different outputs based on the shape being drawn.
Using inheritance, C# developers can create hierarchical class structures that reflect real-world relationships, improve code clarity, and enhance the object-oriented nature of their applications.
For instance, you might have a base class Animal
with method like Eat()
. Then, subclass like Dog
can inherit this method without redefining them. In .NET, C# supports single inheritance, meaning a class can inherit from only one other class.
Base and Derived Classes:
public class Animal
{
public void Eat() { /* implementation here */ }
}
public class Dog : Animal
{
public void Bark() { /* implementation here */ }
}
In this case, Dog
is a derived class, and it inherits the Eat
method from the Animal
base class. Thus, an object of the Dog
class can both Eat
and Bark
.
The base
Keyword: Used to call a method or constructor in the base class from the derived class.
Polymorphism
Polymorphism, a core tenet of object-oriented programming in C#, allows treating objects as instances of their parent class instead of their actual derived class. This enables a single function to take on many forms. In simpler terms, polymorphism lets you interact with several related types in a uniform manner.
There are two primary types of polymorphism in C#:
- Compile-Time Polymorphism (also known as method overloading): This occurs when multiple methods have the same name but different parameters. It’s like a kitchen appliance that can perform different functions (e.g., blend, chop, mix) depending on what button you press.
- Run-Time Polymorphism (also known as method overriding): This happens when a method in a derived class has the same signature as a method in the base class. Here, the derived class provides a specific implementation of the method that overrides the base class implementation.
Here are three specific benefits of polymorphism in C#:
- Flexibility: Polymorphism gives you the flexibility to call the same method on different objects and have each of them respond in their way. For example, you could have a single method
Draw()
that, when called on different shape objects (Circle
,Square
,Triangle
), draws the appropriate shape. - Simplicity: It simplifies code management by allowing you to write more general and abstract code. Instead of having separate methods for each object type, you can write code that works with the general base class.
- Maintainability: When you need to make changes, polymorphism helps isolate them. If you need to modify an object’s behavior, you often only need to change it in one place. This can reduce the chance for errors, making your codebase more robust and easier to maintain.
In C#, polymorphism often involves using virtual
and override
keywords for method overriding, and new
keyword for method hiding. This allows derived classes to provide a specific implementation for a method their base class has already defined.
For instance, both a Cat
and a Dog
class could inherit from an Animal
class and have their own implementation of a Speak()
method.
public class Animal
{
public virtual void Speak()
{
Console.WriteLine("Animal makes a sound");
}
}
public class Cat : Animal
{
public override void Speak()
{
Console.WriteLine("Meow");
}
}
public class Dog: Animal
{
public override void Speak()
{
Console.WriteLine("Woof");
}
}
By marking the base method with the virtual
keyword, it allows derived classes to override it with their own implementation using the override
keyword.
Abstraction
Abstraction in C# is a concept that involves hiding complex reality while exposing only the necessary parts. It’s a way to manage complexity by allowing you to think about problems at a higher level without needing to understand all the details at once.
In C#, you often achieve abstraction using abstract classes and interfaces:
- Abstract Classes: You can create a class that must be inherited by other classes and cannot be instantiated on its own. This class can define abstract methods that lack a body and that derived classes must implement. For example, you might have an abstract class
Vehicle
that has an abstract methodMove()
. The specific way aCar
moves, which is different from how aBicycle
moves, is implemented in their respective derived classes. - Interfaces: An interface is a contract that defines a set of methods without implementing them. Classes that implement the interface agree to implement all the methods defined by the interface, thereby adhering to the contract. For example, an
IDriveable
interface might require any class that implements it to have aDrive
method.
An abstract class, serving as a base for other classes, cannot be instantiated. Let’s see example below:
public abstract class Shape
{
public abstract double Area();
}
public class Circle : Shape
{
public double Radius { get; set; }
public override double Area()
{
return 3.14 * Radius * Radius;
}
}
In the example, Shape
has an abstract method Area()
which means every derived class, like Circle
, must provide an implementation for it.
Additional Concepts
- Interfaces: An interface defines a contract. Any class that implements an interface must provide an implementation of its members. Unlike inheritance, in C#, a class can implement multiple interfaces.
- Composition: It’s a design principle where a class comprises one or more objects from other classes instead of inheriting from them. Developers often favor this over inheritance because it offers more flexibility.
- Delegates and Events: Delegates are a type-safe way to define and manipulate callbacks. Events, based on delegates, allow a class to notify other classes when something of interest occurs.
Advantages of OOP in C#
- Reusability: Inheritance allows for the reuse of existing code, reducing redundancy.
- Scalability: It’s easier to add new features or make changes.
- Maintainability: Code structured around objects is often more readable and easier to maintain.
- Security: Encapsulation keeps data safe, exposing it only when necessary.
Understanding Classes and Objects
Knowing about C# Classes and Objects is very important in object-oriented programming (OOP). They serve as the basic elements of OOP and help in creating complex software systems. Understanding Classes and Objects enables programmers to design and write code for repeated use. This helps in creating software that is easy to change, can handle large amounts of work, and is easy to maintain. This leads to better productivity and efficiency in software development projects.
What is a Class?
A class in C# is like a blueprint for creating objects, a core concept in the object-oriented programming paradigm. Imagine you’re an architect designing a house: the blueprints define the structure and features of the houses you will build, but they are not houses themselves. Similarly, a class defines the structure and behaviors (properties and methods) that objects created from it will possess.
Here are three specific benefits of using classes in C#:
- Organization: A class organizes data and behavior in one place. It’s like having a dedicated folder for all documents related to a specific project. This makes your code cleaner, easier to understand, and manage.
- Reusability: Once you’ve defined a class, you can create as many objects (known as instances) as you need without rewriting code. It’s like using the same blueprint to build multiple houses in a neighborhood, saving time and effort.
- Inheritance: Classes support inheritance, meaning you can create new classes that are based on existing ones. This is like designing a new house model that includes all the features of an existing model plus some additional ones. It promotes code reuse and can simplify the maintenance and expansion of your codebase.
Classes are the cornerstone of C# object-oriented programming, enabling the modeling of complex behaviors and the creation of sophisticated, well-organized, scalable, and maintainable programs.
Components of a Class
A class in C# comprises several components that work together to define its characteristics and capabilities. These components allow a class to encapsulate data and provide functionality. Here are the primary components of a class in C#:
- Fields: Variables declared within a class represent the state or data of the objects created from the class. Think of fields as the characteristics of the class, such as the color or size in a class representing a T-shirt.
- Properties: Properties are a combination of methods that allow controlled access to the class’s fields. If fields are like private diary entries, properties are like reading a summarized, edited version that’s safe to share. They can enforce rules for accessing and updating values, helping to maintain integrity and security of the data.
- Methods: Methods are blocks of code that define actions or behaviors the class can perform. Like a recipe, a method provides the steps needed to accomplish a specific task. When you call a method, you’re telling the object to execute the steps in the recipe.
- Constructors: Special methods called constructors activate when creating a new instance of a class. Constructors typically initialize the fields of the class to default values. It’s like setting up a new phone for the first time, ensuring it’s ready to use.
- Events: Events are a way for a class to provide notifications. A class can raise them to signal that something has happened. Like a doorbell that announces visitors, events let other parts of the program know that something of interest has occurred.
- Destructors: Rarely used in C#, destructors are methods that activate when the system is about to destroy an instance of a class. They typically serve to clean up resources if needed, though the .NET Framework’s garbage collector usually manages this automatically.
- Nested Classes: These classes exist as declarations within another class. A nested class can handle some aspects of the containing class’s functionality that might be cumbersome to implement directly within the containing class.
Together, these components allow a C# class to encapsulate data, manage complexity, and promote code reuse, making the class a powerful construct for creating objects in object-oriented programming.
Encapsulation and Abstraction
A class embodies the principle of encapsulation, where it bundles data (attributes) and methods that operate on that data into a single unit. This not only ensures data integrity but also provides abstraction. Abstraction means exposing only the necessary features of an object and hiding the internal mechanics.
Blueprint Analogy
Consider a class as a blueprint for constructing a building. The blueprint itself isn’t a building but defines how to construct a building. In the same vein, a class isn’t an actual object but outlines how to structure and dictate an object’s behavior. When someone constructs a building based on the blueprint, it becomes an instance of that blueprint. Similarly, when someone creates an object from a class, we say it’s an instance of that class.
Static vs. Instance Members
In C#, you can categorize class members into two main types: static members and instance members. Understanding the difference between these two is crucial for designing and implementing classes properly in object-oriented programming.
Instance Members:
Instance members belong to an instance of a class. Each object or instance of the class has its own copy of these members. Instances do not share them. To access an instance member, you must first create an object of the class.
Benefits of instance members:
- Individual State: Each instance can maintain its own state through instance variables. For example, if you have a
Car
class, eachCar
object can have a differentcolor
orspeed
. - Object-specific Behavior: Methods that operate on the instance variables can produce behavior unique to the particular object. This allows one
Car
toStart()
orStop()
independently of otherCar
objects. - Dynamic Allocation: When you create a new object, it dynamically allocates instance members, and they can be garbage-collected when no longer in use, which helps in efficiently managing memory.
Static Members:
Static members belong to the class itself rather than any particular instance. They are shared across all instances of the class. You access static members using the class name instead of an object reference.
Benefits of static members:
- Shared Resources: Use static members for values or behaviors that all instances of a class should share, such as a counter tracking the number of objects created from a class.
- Utility Functions: Sometimes, a class might provide functionality that doesn’t require an object’s state. For such cases, static methods can be used, such as
Math.Sqrt()
, which is a static method of theMath
class. - Constants and Configuration: Static variables can be used to define constants or configuration settings that are consistent across all instances of the class, like a
PI
constant in theMath
class.
In practice, you might find a class that contains both static and instance members. The decision to make a member static or instance hinges on whether the member’s data or behavior is specific to individual objects created from the class or common to all instances.
Defining a Class in C#
Here’s a simple class definition for a Person
:
public class Person
{
// Fields or Member Variables
private string name;
private int age;
// Properties
public string Name
{
get { return name; }
set { name = value; }
}
public int Age
{
get { return age; }
set { age = value; }
}
// Methods
public void IntroduceYourself()
{
Console.WriteLine($"Hello, I'm {name} and I'm {age} years old.");
}
}
In this class:
name
andage
are fields that store data.Name
andAge
are properties that provide a mechanism to read, write, or compute the values of private fields.IntroduceYourself
is a method that defines a behavior.
What is an Object?
In C# object-oriented programming, an object acts as a fundamental building block of applications, representing an instance of a class. A class provides a blueprint for objects, defining the properties and behaviors that the instantiated objects will have. Creating an object from a class brings together a collection of data (fields, properties) and methods (functions, procedures) into a single unit that you can manipulate within your code.
The concept of an object in C# allows programmers to create modular, reusable, and organized code. Objects can interact with one another, which enables the creation of complex systems through simple, interacting components. This is the essence of object-oriented programming: to model real-world entities and relationships in a way that makes it easier to build, maintain, and evolve software systems.
Characteristics of an Object
In C#, as in other object-oriented programming languages, objects have several defining characteristics:
- Identity: Each object possesses a unique identity that allows you to distinguish it from other objects. Even when two objects share the same state (data values), they remain separate entities. In C#, an object’s identity typically comes from its memory address in the heap where the system allocates it.
- State: The values of fields (also known as properties or attributes) represent an object’s state at any given time. An object’s state can change over time, usually in response to method calls. For instance, if you have a
LightBulb
object, its state may include fields likeisOn
to indicate whether the light is on or off, andcolor
to indicate the color of the light. - Behavior: Methods inside the class define the behavior of objects. These methods usually manipulate the object’s state and can perform tasks. The behavior of a
Car
object, for example, might include methods likeStartEngine()
,StopEngine()
,Accelerate()
, andBrake()
. - Encapsulation: This is the practice of keeping the state and behavior of an object hidden from the outside world. In C#, this is achieved using access modifiers such as
private
,protected
, andpublic
. Encapsulation enables an object to conceal its internal state and demands that all interactions occur through its methods, thus offering a controlled interface to the outside world. - Lifespan: Objects go through a lifespan; you can create, use, and then discard them. In C#, objects are created using the
new
keyword and they live in the managed heap. When they are no longer referenced by any part of your program, they become eligible for garbage collection, at which point their resources are reclaimed by the runtime. - Relationships: Objects can have relationships with other objects. You can categorize these relationships as associations (plain interaction), aggregations (a whole-part relationship where the part can exist independently of the whole), and compositions (a whole-part relationship where the part cannot exist independently of the whole).
- Polymorphism: Objects can take on more than one form depending on the context. In C#, polymorphism allows methods to do different things based on the object’s actual derived type that is being referenced.
- Inheritance: Objects can inherit the state and behavior from other objects. In C#, you accomplish this by creating derived classes that inherit from a base class. This allows for code reuse and the creation of more complex behaviors based on simple building blocks.
These characteristics help to promote flexible, modular, and reusable code, which are hallmarks of object-oriented programming.
Creating Objects in C#
We call the creation of an object is instantiation. In C#, this is typically done using the new
keyword followed by the class constructor. For example, consider a class called Person
. The Person
class might define properties like Name
and Age
, and method such as IntroduceYourself()
. An object is then created from this class, often using the new
keyword, and it encapsulates all these attributes and behaviors. So, when you write:
Person johnDoe = new Person();
Here, johnDoe
is an object of the Person
class. We used the new
keyword to instantiate the object. Each object maintains its own state, so if you create multiple Person
objects, each can have its own values for Name
and Age
.
Features of Working with Objects
Working with objects in C# involves creating instances of classes to utilize their defined properties and methods. This concept is integral to C#’s object-oriented nature, providing several features:
- Lifecycle: Objects have a lifecycle. They are created, they can undergo state changes, and eventually, they can be destroyed. In C#, object destruction and memory cleanup are managed by the garbage collector when no references to the object remain.
- Accessing Object Members: Once an object is created, you can interact with its members (both fields and methods) using the dot (
.
) operator.
johnDoe.Name = "John Doe";
johnDoe.Age = 30;
johnDoe.IntroduceYourself(); // Call a method on the 'johnDoe' object
- Object References: In C#, objects are reference types. This means when you assign one object to another, both variables point to the same object in memory. Any changes one variable undergoes will reflect in the other.
Person anotherReference = johnDoe;
anotherReference.Name = "Jane";
Console.WriteLine(johnDoe.Name); // Outputs "Jane"
- Static Members: A class can have static fields, properties, and methods. These belong to the class itself, rather than to any specific object. For instance, if you wanted to track how many
Person
objects have been created, you could use a static field.
public class Person
{
private static int count = 0;
public Person()
{
count++;
}
public static int Count
{
get { return count; }
}
}
With this, Person.Count
would give you the number of Person
objects created.
Constructors and Destructors
Understanding constructors and destructors is crucial for mastering C# object-oriented programming concepts. They play a crucial role in managing the life cycle of an object, ensuring correct initialization and cleanup. In this chapter, we will delve into their definitions, purposes, intricacies, and best practices to gain a comprehensive understanding of their significance in C# programming.
Constructors
In C#, constructors play a crucial role in the life of an object, and they come with a host of benefits that streamline the creation and initialization of objects. First and foremost, constructors offer a clear and unambiguous method for setting up your objects. When you create a new instance of a class, the constructor assigns values to all the necessary properties, ensuring a well-defined state. This can prevent bugs that occur from undefined or improperly initialized fields.
Secondly, constructors enhance code readability. Encapsulating the initialization logic within a designated method makes it easier for someone reading the code to understand how to set up an object. This can be especially beneficial when working in team environments or when you return to your code after some time.
Lastly, you can overload constructors, allowing multiple constructors with different parameters. This flexibility allows you to create objects in various states, catering to different scenarios. For instance, you could use one constructor to set up a default object and another to accept specific parameters for creating a customized object, offering versatility in initializing class instances.
Characteristics of Constructors:
- Name: A constructor has the same name as its class.
- No Return Type: Constructors don’t have a return type, not even
void
. - Access Modifiers: Like methods, constructors can have access modifiers (e.g.,
public
,private
).
Types of Constructors
- Default Constructor: This constructor doesn’t take any parameters. If no constructor is provided in the class, C# automatically provides a default, parameterless constructor.
public MyClass()
{
// Initialization code here
}
- Parameterized Constructor: Takes parameters to initialize the object’s state.
public MyClass(int initialValue)
{
this.someField = initialValue;
}
- Copy Constructor: Creates an object by copying variables from another object.
public MyClass(MyClass another)
{
this.someField = another.someField;
}
- Static Constructor: It initializes static members of the class and automatically calls before creating the first instance or referencing any static members.
static MyClass()
{
// Initialization of static members
}
Constructor Chaining
In C#, it’s possible to call one constructor from another using the : this()
syntax. This is useful to avoid repeating code across multiple constructors.
public MyClass() : this(0) // Calls parameterized constructor
{
}
public MyClass(int value)
{
this.someField = value;
}
Destructors
Destructors in C# act as a safety net for memory management, ensuring that your program properly releases resources back to the system. Imagine a destructor like a responsible cleaner who comes in after a theater show; it helps clean up once the performance is over. This means that your application is more efficient and doesn’t leave unnecessary data taking up space.
Secondly, destructors are automatic; you don’t need to manually invoke them. Just as household appliances turn off when their timer ends, a destructor automatically activates to dispose of an object when it’s no longer needed, reducing the programmer’s workload and minimizing the risk of forgetting to free resources.
Lastly, destructors are particularly useful when dealing with non-managed resources, such as file handles or database connections. These resemble rented tools that you must return once you’re finished with them. The destructor takes care of this return, preventing issues like data corruption or security holes that could arise from leaving such resources unattended.
Characteristics of Destructors
- Naming: A destructor has the same name as its class but is prefixed with a
~
(tilde). - No Parameters or Modifiers: Destructors cannot have parameters or access modifiers.
- Automatic Invocation: It’s automatically invoked when the object is eligible for garbage collection. You cannot call a destructor explicitly.
public class MyClass
{
// Constructor
public MyClass()
{
// Initialization code here
}
// Destructor
~MyClass()
{
// Cleanup code here
}
}
Important Points about Destructors
- Finalize Method: Internally, the destructor is translated to a
Finalize
method override in the IL (Intermediate Language). - Garbage Collection: Destructors are called non-deterministically by the garbage collector, so you can’t predict exactly when they’ll be executed.
- Dispose Pattern: In practice, the
IDisposable
interface and theDispose
method are preferred for cleanup over destructors because they offer more control and deterministic cleanup of resources.
In conclusion, constructors and destructors play a pivotal role in the lifecycle of an object in C#. The garbage collector calls destructors non-deterministically, so predicting their exact execution time is not possible. Instead, the Dispose
pattern is often employed for resource cleanup.
Properties and Encapsulation
Properties and encapsulation are important concepts in C# and Object-Oriented Programming (OOP). They help manage and protect the state of an object, ensuring data integrity and controlled access to its variables. Properties allow developers to get and set values of an object’s private fields, making it safer to modify the object’s state. Encapsulation combines data and methods in a class, hiding implementation details from the outside. This promotes code reusability, maintainability, and reduces the risk of unintended changes to the object’s state. Let’s now explore these concepts and their practical applications in C# and OOP.
Properties in C#
In C#, properties are a natural evolution of fields (class variables) and provide a mechanism to read, write, or compute the value of a private field. Instead of using getter and setter methods as in some other languages, C# uses properties to provide controlled access to an object’s attributes.
Characteristics of Properties
- Access Modifiers: Like methods, properties can have access modifiers like
public
,private
,protected
, etc. - Get and Set Accessors: Properties have two accessors:
get
(to read the value) andset
(to write a value). You can make a property read-only by providing only aget
accessor, or write-only with only aset
accessor (though this is less common).
Simple Properties
private string _name;
public string Name
{
get { return _name; }
set { _name = value; }
}
Here, the Name
property provides access to the private field _name
. External classes can get or set the Name
without directly accessing _name
.
Auto-Implemented Properties
C# provides a shorthand for properties when no additional logic is required in the get
and set accessors.
public string Name { get; set; }
The compiler automatically creates a private, anonymous field accessible only through the property.
Properties with Logic
You can add logic to properties. For example, to ensure a Person
object’s age is always non-negative:
private int _age;
public int Age
{
get { return _age; }
set
{
if (value >= 0)
_age = value;
else
throw new ArgumentException("Age cannot be negative");
}
}
Computed Properties
Properties don’t necessarily need to back onto a field. They can compute a value based on other data within the class.
public class Rectangle
{
public double Width { get; set; }
public double Height { get; set; }
public double Area
{
get { return Width * Height; }
}
}
In this example, the Area
property calculates the area of the rectangle on-the-fly based on its width and height.
Expression-bodied Properties
Starting with C# 6, you can write properties using lambda-like expressions for more concise definitions:
public double Area => Width * Height;
Property Initializers
From C# 6 onwards, you can also initialize auto-implemented properties similarly to fields:
public string Name { get; set; } = "Default Name";
Encapsulation
Encapsulation is one of the four primary pillars of object-oriented programming (along with inheritance, polymorphism, and abstraction). It refers to the bundling of data (attributes) and methods (functions) that operate on the data into a single unit, i.e., the class, while restricting direct access to some of the object’s components. Encapsulation ensures that:
- An object’s internal state shields itself from direct manipulation from outside.
- The object hides implementation details, presenting a clear and consistent external interface.
- It helps to achieve a separation of concerns.
Importance of Properties in Encapsulation
Properties are central to the principle of encapsulation for several reasons:
- Validation: As seen in the
Age
property example, you can add validation logic to ensure the object always remains in a valid state. - Flexibility: Even if the internal implementation changes, the external interface (the property) remains consistent. This means other parts of the code that interact with the object don’t need to change.
- Protection: By encapsulating fields behind properties, you can protect the inner state of your object. For instance, you can make the
set
accessorprivate
if you only want the field to be modified internally within the class.
Encapsulation in Practice
1. Read-only Properties
In some scenarios, you may want to set certain class properties, like an ID, only once. In such cases, you can leverage read-only properties:
public class Person
{
private readonly string _id;
public Person(string id)
{
_id = id;
}
public string ID => _id;
}
2. Full vs. Backing Properties
You might wonder why one might use a full property with a backing field over a simpler auto-implemented property. The key reason is to insert logic in the property accessors. A full property provides flexibility to add custom logic during the get/set operations, which is not possible with auto-implemented properties.
3. Private Setters
While you can make a property public, you can restrict its setter:
public string Name { get; private set; }
Here, external entities can read the Name
but cannot modify it. Only methods within the class can change its value.
4. Property Change Notifications
In applications, especially UI-driven ones, there might be a need to notify other parts of the system when a property’s value changes. The .NET framework provides the INotifyPropertyChanged
interface to achieve this:
public class ObservablePerson : INotifyPropertyChanged
{
private string _name;
public string Name
{
get { return _name; }
set
{
if (_name != value)
{
_name = value;
OnPropertyChanged(nameof(Name));
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
With this pattern, any subscriber can be notified of property changes and react accordingly, commonly used in data binding scenarios in UI frameworks like WPF or Xamarin.
5. Encapsulation Beyond Properties
While properties are a primary tool for encapsulation in C#, it’s worth noting that encapsulation applies to all members of a class. You can encapsulate methods, events, and even other classes or structures (nested types) using access modifiers and design patterns.
For instance, exposing a method that allows controlled manipulation of internal data, while keeping the actual data structure (like a list) private, is a form of encapsulation:
private List<string> _items = new List<string>();
public void AddItem(string item)
{
// Some validation or logic here
_items.Add(item);
}
public IEnumerable<string> GetItems()
{
return _items.AsReadOnly();
}
In essence, properties are a robust tool in C# to achieve encapsulation. They enable controlled access and manipulation of an object’s state, ensuring that objects adhere to specific behaviors, remain in valid states, or notify the system of internal changes. Properties, combined with other OOP principles and .NET features, play a pivotal role in building resilient and maintainable software architectures in C# object-oriented programming.