Do you think you fully understand Object-Oriented Programming in C#? Think again! Many developers, even experienced ones, miss crucial nuances that can elevate their code from good to great. Today, we’ll uncover those hidden gems together!
If you’ve ever felt like you’re writing endless classes but not getting the full power of OOP in C#, this article is exactly what you need. We’re going to walk step by step through the core principles of OOP — and I’ll show you real-world examples from my own projects. No abstractions, just practical insights you can use right away.
Understanding Object-Oriented Programming (OOP)
Before we dive into C# specifics, let’s refresh what OOP is. In simple terms, Object-Oriented Programming is a paradigm where software design revolves around objects. Each object represents a real-world entity with its own state (properties) and behavior (methods).
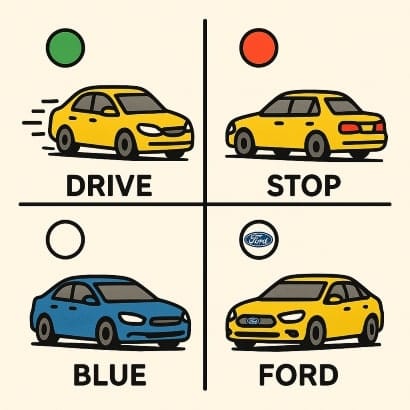
Main Advantages:
- Reusability: Write once, use multiple times. No need to duplicate code across different parts of your application.
- Maintainability: Easier to manage and update code. Changes in one place ripple effectively across all uses.
- Scalability: Build systems that grow easily with new requirements without rewriting existing logic.
- Real-world Mapping: Models real scenarios naturally. For example, a “Car” object in your program mirrors an actual car’s properties and actions.
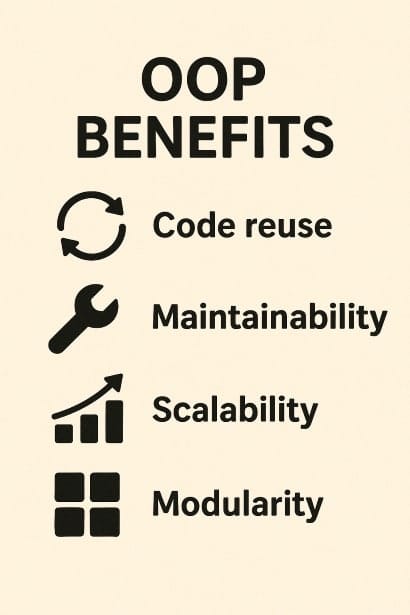
In C#, you unlock the full power of OOP by leveraging classes, objects, interfaces, inheritance, and more. Now, let’s dive deeper into each concept.
Core Concepts of OOP in C#
Classes and Objects
Think of a class as a blueprint, and an object as the actual house you build from that blueprint. Classes define the structure, and objects are instances that bring the structure to life.
public class Car
{
public string Brand { get; set; }
public int Year { get; set; }
public void Drive()
{
Console.WriteLine($"Driving {Brand} made in {Year}");
}
}
// Creating an object
Car myCar = new Car { Brand = "Tesla", Year = 2024 };
myCar.Drive();
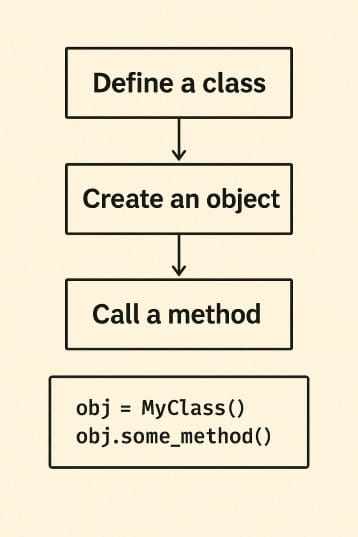
Practical tip: Use constructors to simplify object initialization and enforce required properties at the time of object creation.
public Car(string brand, int year)
{
Brand = brand;
Year = year;
}
This ensures your objects are always in a valid state.
Inheritance
Inheritance allows you to create a new class based on an existing one. It promotes code reusability and hierarchical relationships, making your code cleaner and easier to maintain.
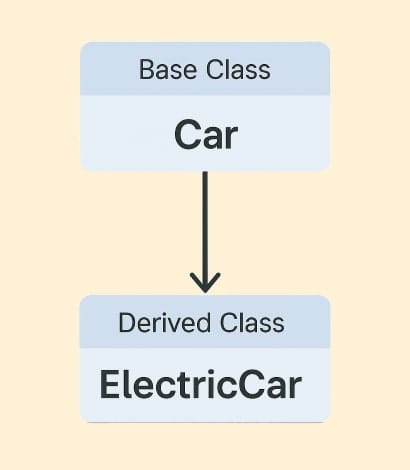
public class ElectricCar : Car
{
public int BatteryCapacity { get; set; }
public void Charge()
{
Console.WriteLine("Charging the electric car...");
}
}
ElectricCar tesla = new ElectricCar { Brand = "Tesla", Year = 2024, BatteryCapacity = 100 };
tesla.Drive();
tesla.Charge();
In my projects, inheritance saves tons of time when implementing shared behaviors! However, be cautious of the “deep inheritance tree” anti-pattern — too many layers of inheritance can make debugging and maintenance challenging.
Encapsulation
Encapsulation is about data protection. You hide internal state and expose only what’s necessary. This is key to building secure and maintainable systems.
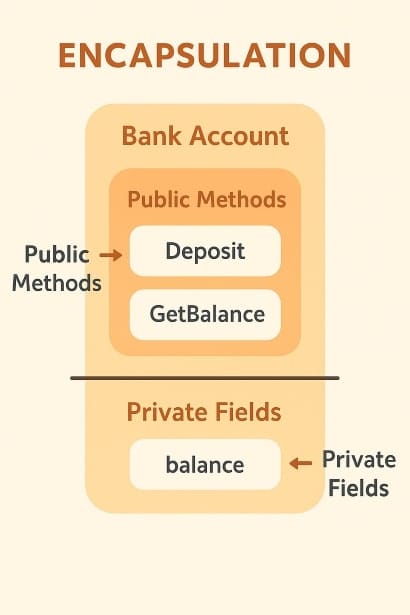
public class BankAccount
{
private decimal balance;
public void Deposit(decimal amount)
{
if (amount > 0)
balance += amount;
}
public decimal GetBalance()
{
return balance;
}
}
BankAccount account = new BankAccount();
account.Deposit(500);
Console.WriteLine(account.GetBalance());
Use access modifiers (private
, public
, protected
, internal
) wisely to control the visibility of your class members. A well-encapsulated class is like a well-secured vault — it keeps unwanted access at bay.
Polymorphism
Polymorphism allows objects to be treated as instances of their parent class rather than their actual class, providing flexibility in code execution.
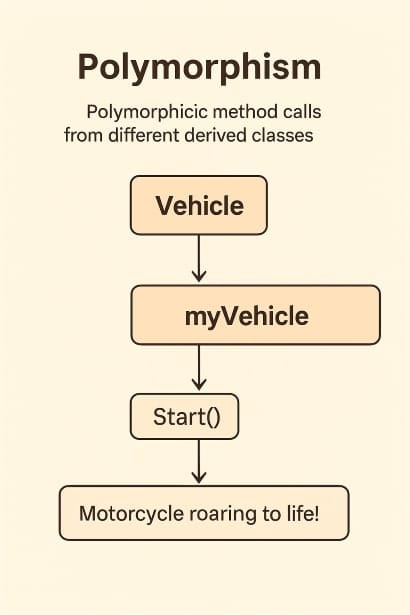
public class Vehicle
{
public virtual void Start() => Console.WriteLine("Vehicle starting...");
}
public class Motorcycle : Vehicle
{
public override void Start() => Console.WriteLine("Motorcycle roaring to life!");
}
Vehicle myVehicle = new Motorcycle();
myVehicle.Start();
Abstraction
Abstraction means exposing only essential features while hiding the complex details. This is the art of simplifying complexity!
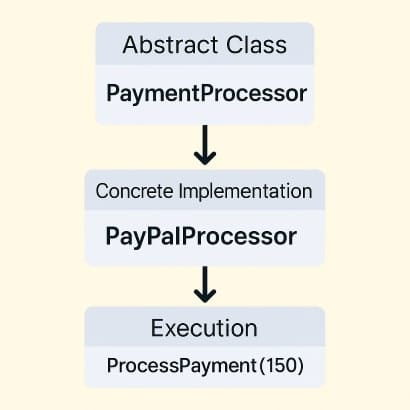
public abstract class PaymentProcessor
{
public abstract void ProcessPayment(decimal amount);
}
public class PayPalProcessor : PaymentProcessor
{
public override void ProcessPayment(decimal amount)
{
Console.WriteLine($"Processing ${amount} through PayPal.");
}
}
PaymentProcessor processor = new PayPalProcessor();
processor.ProcessPayment(150);
Abstract classes and interfaces allow you to define contracts for your code, ensuring consistency and flexibility across implementations. I often use abstraction to define service layers in enterprise applications, separating the business logic from the infrastructure.
FAQ: Clarifying Common Doubts About OOP in C#
No. Use inheritance only when there’s a clear “is-a” relationship. For more flexible designs, consider composition or interfaces.
They are related but different. Encapsulation hides data, while abstraction hides complexity. Both promote cleaner code but serve different purposes.
Interfaces define a contract without implementation. They are essential for dependency injection, mocking in unit tests, and building flexible systems.
C# doesn’t support multiple class inheritance but allows multiple interface implementations. This is a safer and cleaner approach to combining behaviors.
Conclusion: Mastering OOP in C# is Your Next Power Move!
We just unpacked the essential OOP concepts in C#, but the journey doesn’t stop here. Every real-world application of these principles will sharpen your skills. I encourage you to revisit your projects and spot areas where you can apply encapsulation, abstraction, or even rethink inheritance!
Remember, the true mastery of OOP isn’t just knowing the definitions — it’s about applying them thoughtfully in your daily coding. Refactor an old class, design a cleaner interface, or try implementing a design pattern using these principles.
If you found this guide helpful, drop your thoughts in the comments. Have questions or want me to cover interfaces and dependency injection next? Let me know!
- Introduction to C# Development for Beginners
- .NET Dev Environment Setup Made Easy
- Key OOP Concepts in C#: Classes, Inheritance, Polymorphism
- Basic C# Syntax and Your First Program
- C# Data Types Guide 2025 – Fast, Safe, Precise