LINQ (Language Integrated Query) is like the Swiss Army knife of .NET. Need to query collections? Done. Manipulate data? Easy. Process sequences? Child’s play. LINQ gives developers a fluent way to handle data directly in C# and VB.NET. But let’s be real: as data grows messier and logic gets gnarlier, vanilla LINQ starts feeling a bit… limited.
Enter MoreLINQ, the open-source extension that takes LINQ from good to legendary. Built by the community, for the community, MoreLINQ expands LINQ’s repertoire with advanced operators that make complex queries feel like a breeze. Think of it as unlocking “God Mode” for your data manipulations.
What is MoreLINQ?
MoreLINQ is an open-source library that supercharges LINQ with dozens of additional methods designed to tackle advanced scenarios. It started as a passion project by Jon Skeet (yes, the Jon Skeet) and has since blossomed into a robust, community-maintained package.
- History & Community: Born out of real-world frustrations with LINQ’s limitations.
- Where to find it?
- GitHub: MoreLINQ on GitHub
- NuGet: Installable via NuGet for smooth integration.
- Maintained actively, ensuring you always have up-to-date enhancements at your fingertips.
Why Developers Use MoreLINQ
Let’s get straight to the point: standard LINQ is awesome, but it doesn’t cover everything.
Common LINQ Limitations:
- Lack of advanced operators (like batching or advanced filtering).
- Complex chaining gets unreadable.
- Custom solutions for common problems waste time.
MoreLINQ to the rescue:
- Advanced operators: Cleaner, faster, more readable.
- Performance boosts: Reduces boilerplate, optimizes common tasks.
- Real-world use cases: Data pipelines, aggregations, and bulk operations.
MoreLINQ makes complex data manipulations feel natural. Imagine slicing large datasets into manageable chunks or filtering lists by specific properties with just one line of code.
Key Features and Extensions
Let’s dive into the good stuff!
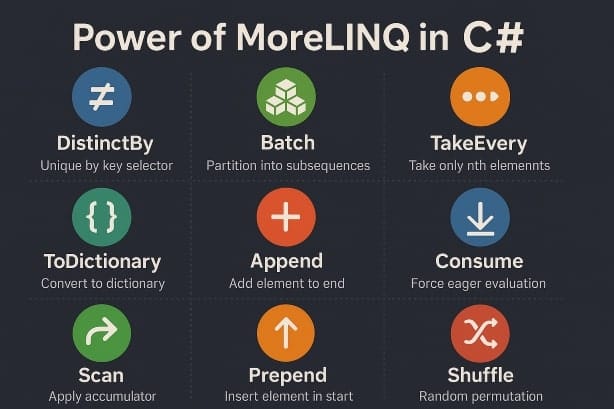
Batching and Buffering
Need to process data in batches? Use Batch()
:
var numbers = Enumerable.Range(1, 10);
var batches = numbers.Batch(3);
foreach (var batch in batches)
{
Console.WriteLine(string.Join(", ", batch));
}
Result:
1, 2, 3
4, 5, 6
7, 8, 9
10
Explanation: Processes data in fixed-sized groups, great for pagination or bulk processing.
DistinctBy
Skip manual grouping! Use DistinctBy()
:
var people = new[]
{
new { Name = "Alice", Age = 30 },
new { Name = "Bob", Age = 40 },
new { Name = "Alice", Age = 25 }
};
var uniqueByName = people.DistinctBy(p => p.Name);
Result: Only the first occurrence of “Alice” and “Bob” remain.
Explanation: Eliminates duplicates based on a selected property.
Generate and Repeat
Generate sequences effortlessly:
var sequence = MoreEnumerable.GenerateByIndex(index => index * 2).Take(5);
foreach (var number in sequence)
{
Console.WriteLine(number);
}
Result:
0
2
4
6
8
Explanation: Creates custom sequences with predictable patterns.
Scan and Aggregate Operations
Go beyond Aggregate()
with Scan()
:
var numbers = new[] { 1, 2, 3, 4 };
var runningTotals = numbers.Scan(0, (sum, n) => sum + n);
foreach (var total in runningTotals)
{
Console.WriteLine(total);
}
Result:
0
1
3
6
10
Explanation: Provides running cumulative results, perfect for financial calculations.
Fallback and Default Operations
Prevent errors with FallbackIfEmpty()
:
var emptyList = Enumerable.Empty<int>();
var safeList = emptyList.FallbackIfEmpty(-1);
foreach (var item in safeList)
{
Console.WriteLine(item);
}
Result:
-1
Explanation: Supplies a default value when the sequence is empty.
Installation and Setup
Getting started is super easy!
- Install via NuGet:
dotnet add package MoreLinq
- Basic Setup: Just import the namespace:
using MoreLinq;
- Tips for Integration:
- Use alongside existing LINQ queries for seamless enhancement.
- No major refactoring needed.
Best Practices for Using MoreLINQ
- Use when needed: Avoid replacing simple LINQ queries with MoreLINQ just for the sake of it.
- Performance matters: Some operators process in-memory data, so use wisely for large datasets.
- Maintain readability: Favor clarity over cleverness to keep your codebase maintainable.
- Combine smartly: Use MoreLINQ with Entity Framework carefully—ensure queries are evaluated in memory where required.
Real-World Use Cases
- Data processing pipelines: Batch processing millions of records efficiently.
- Reporting and Aggregation: Create dynamic reports using
Scan()
andAggregate()
. - Business logic readability: Replace convoluted loops with expressive, declarative MoreLINQ methods.
FAQ: Common Questions About MoreLINQ
Nope, it extends LINQ. Think of it as LINQ’s cool, older sibling.
Generally negligible, but watch out for in-memory processing.
Partially! Some operators need to run in-memory post-fetching data.
Conclusion: Supercharge Your LINQ with MoreLINQ
As we’ve explored, MoreLINQ is not just an add-on but a power-up for your everyday LINQ queries. From simplifying data manipulations to writing cleaner and more maintainable code.
So, why not give it a try today? Install MoreLINQ, refactor some queries, and experience the clarity and power it brings! And don’t forget to drop a comment sharing your favorite trick!