In the realm of artificial intelligence, the OpenAI DALL-E 2 API stands as a powerful tool that empowers developers and artists to generate creative and imaginative art. This API harnesses the capabilities of deep learning to produce images from textual descriptions. In this post, we’ll delve into the world of OpenAI DALL-E API, explore how it works, and provide hands-on examples of integrating it using the C# programming language.
Understanding OpenAI DALL-E API
OpenAI DALL-E 2 API is built upon advanced deep learning models that have been trained to transform textual prompts into corresponding images. The name “DALL-E” is a fusion of the famous surrealist artist Salvador Dali and Pixar’s lovable character WALL-E, representing the blend of artistic creativity and advanced technology.
The core concept of the DALL-E API revolves around providing textual descriptions as input and receiving image outputs as responses. This process involves encoding the text into a format that the model can understand and then decoding the model’s response into an image.
Getting Started with C# and OpenAI DALL-E API
To work with the OpenAI DALL-E API in C#, you need to make HTTP requests to the API endpoint using libraries like RestSharp
. Here’s a step-by-step guide:
Step 1: API Key
First, obtain your API key from OpenAI. This key authenticates your requests to the DALL-E API.
Step 2. Import Necessary Libraries
using Newtonsoft.Json.Linq;
using RestSharp;
using RestSharp.Authenticators.OAuth2;
using System.Configuration;
using System.Net;
Step 3. Set Up API Key
Replace "YOUR_API_KEY"
into App.config with your actual OpenAI API key.
var apiKey = ConfigurationManager.AppSettings["OpenaiAPIKey"];
var options = new RestClientOptions("https://api.openai.com/v1/images/generations")
{
Authenticator = new OAuth2AuthorizationRequestHeaderAuthenticator(apiKey, "Bearer"),
};
Step 4. Compose the Request
Create a method that prepares the request to send a description to the DALL-E API.
static string GenerateImage(string description)
{
var request = new RestRequest()
{
Method = Method.Post,
RequestFormat = DataFormat.Json
};
var param = new {
prompt = description,
n = 1,
size = "256x256"
};
request.AddJsonBody(param);
return client.Execute(request).Content;
}
The response you receive from the API will contain information about the generated image. The actual image data can be extracted from the JSON response and saved as an image file.
Step 5. Handle the Response
Invoke the method and process the response to get the generated image URL.
static string GetGeneratedImageURL(string response)
{
dynamic jsonResponse = JObject.Parse(response);
return jsonResponse["data"][0]["url"];
}
Step 6. User Interaction
Enhance the user interaction by allowing them to input descriptions and see the generated images.
static void Main(string[] args)
{
Console.WriteLine("Enter a description for the image:");
var description = Console.ReadLine();
var response = GenerateImage(description);
var imageUrl = GetGeneratedImageURL(response);
Console.WriteLine($"Generated Image URL: {imageUrl}");
// Display the image or integrate it into your application
}
Here Some Examples Generated Images:
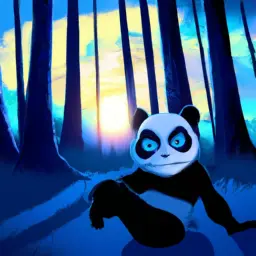
Prompt: Kung fu panda in the blue forest at sunset
Prompt: Smiling cat sitting on a stump
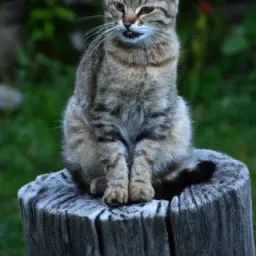
References:
- OpenAI API Documentation: platform.openai.com
- OpenAI API Example Project: OpenAI-Examples
Unleash Your Creativity
The OpenAI DALL-E API opens up exciting possibilities for creating art, generating visuals for various applications, and even aiding in design processes. With a blend of textual prompts and powerful deep learning, developers can now seamlessly integrate imaginative image generation into their projects using C#.
In this post, we explored the concept of the OpenAI DALL-E API, walked through the process of sending requests using C#, and discussed how to handle and utilize the response. Now, armed with this knowledge, you can embark on a journey of creativity and innovation by leveraging the capabilities of the DALL-E API.