Performance is a critical aspect of software development, and optimizing code for speed and efficiency is a common goal for developers. One way to achieve this is through benchmarking, a process that measures the performance of code or algorithms under specific conditions. Benchmarking helps developers identify bottlenecks, compare different implementations, and make data-driven decisions to improve their application’s performance. In the .NET ecosystem, BenchmarkDotNet is a powerful and widely-used benchmarking library that simplifies the process of performance benchmarking in C#.
What is BenchmarkDotNet?
BenchmarkDotNet is an open-source library developed by Andrey Akinshin that facilitates easy and reliable performance benchmarking in C# and .NET. It provides a simple and flexible API to write and execute benchmarks with minimal overhead and accurate results. BenchmarkDotNet is well-suited for benchmarking various aspects of .NET applications, including methods, classes, algorithms, data structures, and much more.
Key Features of BenchmarkDotNet
- Simple and Clear API: BenchmarkDotNet offers an intuitive and straightforward API that allows developers to write benchmarks with ease. The API provides attributes and methods to define benchmarks, iterations, warm-up periods, and target environments.
- Automatic Execution: BenchmarkDotNet automatically handles the repetitive tasks associated with benchmarking, such as warm-up iterations, multiple runs, and statistical analysis of results. It ensures that the benchmarks are executed in a consistent and reliable manner.
- JIT Optimization Suppression: BenchmarkDotNet utilizes a sophisticated infrastructure to ensure the .NET just-in-time (JIT) compiler doesn’t interfere with benchmark results, providing accurate and meaningful performance metrics. This is crucial because the JIT compiler may optimize code differently, depending on how often and when it’s executed.
- Support for Multiple Runtimes: It supports benchmarking on various .NET runtimes, including .NET Framework, .NET Core, and .NET 5 and above. This allows developers to evaluate the performance of their code across different platforms and choose the most performant runtime for their application.
- Customization Options: The library allows customization of benchmark execution, such as specifying the number of iterations, the number of warm-up runs, and the precision of measurements. This level of customization ensures that benchmarks can be tailored to meet specific requirements.
- Powerful Analyzers: BenchmarkDotNet provides detailed reports, including statistical analysis, memory allocation data, and performance comparisons, making it easy to spot performance regressions and improvements. These reports help developers understand the behavior of their code under various scenarios.
Install BenchmarkDotNet
Installing BenchmarkDotNet is straightforward, and you can do it via NuGet, which is the package manager for .NET. Follow the instructions below to install BenchmarkDotNet in your C# .NET project:
Step 1: Create a New C# .NET Project (Optional)
If you haven’t already, create a new C# .NET project using your preferred development environment (Visual Studio, Visual Studio Code, or others).
Step 2: Open Package Manager Console
In Visual Studio, open the “Package Manager Console” by going to `Tools > NuGet Package Manager > Package Manager Console`.
Step 3: Install BenchmarkDotNet Package
In the Package Manager Console, type the following command and press Enter to install BenchmarkDotNet:
Install-Package BenchmarkDotNet
Alternatively, you can also install it using the dotnet CLI. Open a terminal or command prompt and navigate to your project’s folder. Then, run the following command:
dotnet add package BenchmarkDotNet
Step 4: Verify Installation (Optional)
After installing the package, the necessary dependencies will be downloaded and installed automatically. You can verify the installation by checking the `csproj` file of your project. Open the `.csproj` file and look for a line similar to the following:
<PackageReference Include="BenchmarkDotNet" Version="x.x.x" />
The `Version=”x.x.x”` indicates the installed version of BenchmarkDotNet.
Step 5: Start Benchmarking
With BenchmarkDotNet successfully installed in your project, you can now start writing benchmarks using the library. Refer to the following examples in this post for guidance on how to write and execute benchmarks using BenchmarkDotNet.
Note: BenchmarkDotNet requires .NET Standard 2.0 or .NET Core 2.0 and above, so make sure your project targets a compatible version. Additionally, keep in mind that BenchmarkDotNet works best when run in Release mode without the debugger attached, as it ensures more accurate and reliable benchmarking results.
Getting Started with BenchmarkDotNet
Let’s walk through more examples to demonstrate how to use BenchmarkDotNet for benchmarking in C#.
Example 1: Benchmarking a Mathematical Operation
Suppose we want to benchmark the performance of exponentiation using the `Math.Pow` method and a custom implementation of exponentiation. Let’s define our benchmark class:
using System;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
public class ExponentiationBenchmarks
{
private const double BaseValue = 2.0;
private const int Exponent = 10;
[Benchmark]
public double MathPowBenchmark()
{
return Math.Pow(BaseValue, Exponent);
}
[Benchmark]
public double CustomExponentiationBenchmark()
{
return CustomExponentiation(BaseValue, Exponent);
}
private static double CustomExponentiation(double x, int n)
{
double result = 1.0;
for (int i = 0; i < n; i++)
{
result *= x;
}
return result;
}
}
public class Program
{
public static void Main(string[] args)
{
var summary = BenchmarkRunner.Run<ExponentiationBenchmarks>();
}
}
Result of Benchmarking a Mathematical Operation:
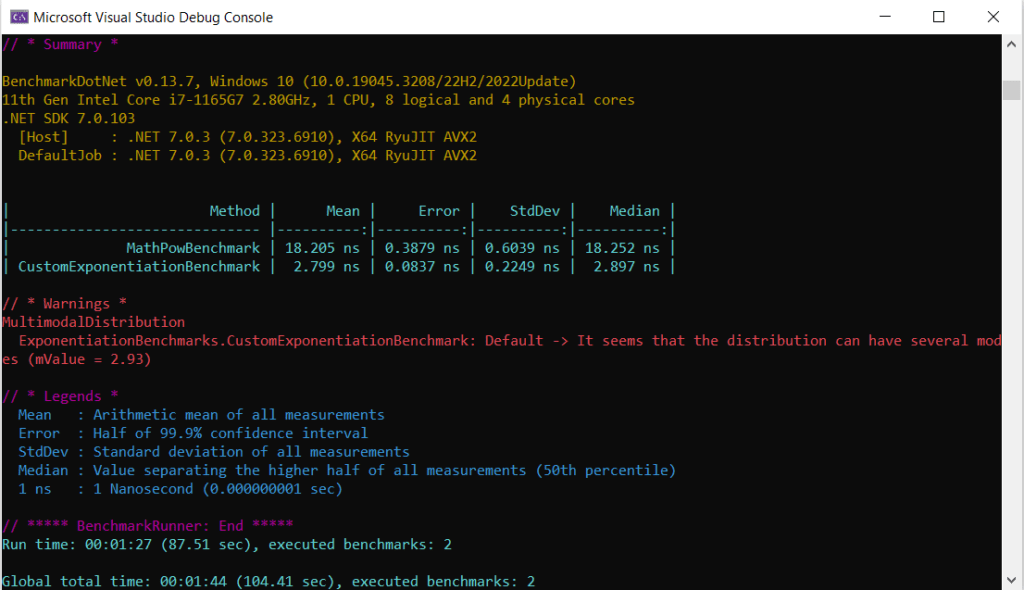
If you observe that CustomExponentiation has a lower mean and median time compared to the built-in Math.Pow method, it suggests that CustomExponentiation method is more efficient for this specific exponentiation operation in the benchmark scenario.
Example 2: Benchmarking Different Sorting Algorithms
Now, let’s benchmark three different sorting algorithms: Bubble Sort, Quick Sort, and Merge Sort:
using System;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
public class SortingBenchmarks
{
private int[] data;
[Params(1000, 10000)]
public int N;
[GlobalSetup]
public void Setup()
{
// Initialize data with random numbers
var random = new Random();
data = new int[N];
for (int i = 0; i < N; i++)
{
data[i] = random.Next();
}
}
[Benchmark]
public void BubbleSort()
{
Array.Sort(data);
}
[Benchmark]
public void QuickSort()
{
// Custom QuickSort implementation
QuickSort(data, 0, data.Length - 1);
}
[Benchmark]
public void MergeSort()
{
// Custom MergeSort implementation
MergeSort(data, 0, data.Length - 1);
}
private void QuickSort(int[] array, int left, int right)
{
// Custom QuickSort implementation
// ...
}
private void MergeSort(int[] array, int left, int right)
{
// Custom MergeSort implementation
// ...
}
}
public class Program
{
public static void Main(string[] args)
{
var summary = BenchmarkRunner.Run<SortingBenchmarks>();
}
}
Result of Benchmarking Different Sorting Algorithms:
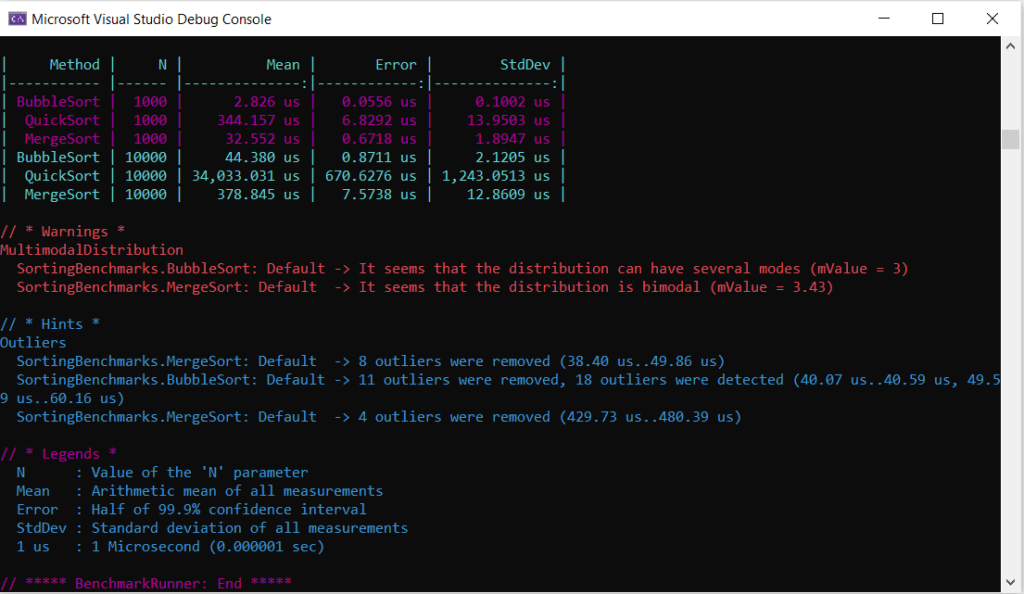
Example 3: Benchmarking a String Comparison
Let’s create an example that benchmarks three different methods for comparing two strings: the built-in `string.Equals` method, a custom method for string comparison and comparing strings using uppercase conversion and the equality operator (`==`). We will compare the performance of these three methods using BenchmarkDotNet.
using System;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
public class StringComparisonBenchmarks
{
private const string SourceString = "Hello, World!";
private const string TargetString = "hello, world!";
[Benchmark]
public bool StringEqualsBenchmark()
{
return string.Equals(SourceString, TargetString, StringComparison.OrdinalIgnoreCase);
}
[Benchmark]
public bool CustomStringComparisonBenchmark()
{
return CustomStringComparison(SourceString, TargetString);
}
[Benchmark]
public bool UpperCaseComparisonBenchmark()
{
return SourceString.ToUpper() == TargetString.ToUpper();
}
private bool CustomStringComparison(string str1, string str2)
{
// Custom case-insensitive string comparison implementation
return string.Compare(str1, str2, StringComparison.OrdinalIgnoreCase) == 0;
}
}
public class Program
{
public static void Main(string[] args)
{
var summary = BenchmarkRunner.Run<StringComparisonBenchmarks>();
}
}
Result of Benchmarking a String Comparison:
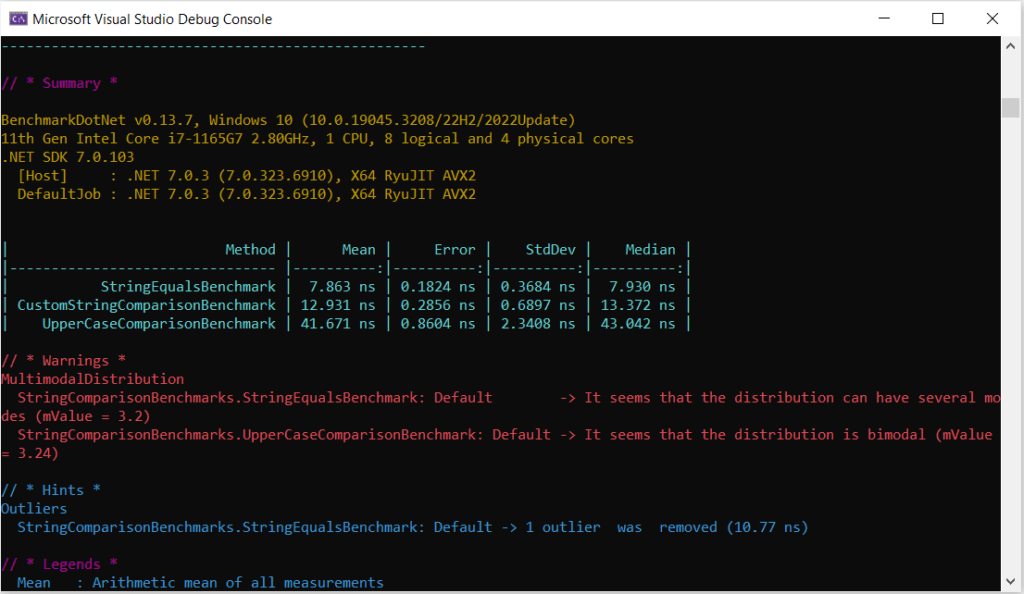
You can find the project with these examples on GitHub using the following link: CSharpBenchmarkDotNetExamples
Running the Benchmarks
To execute the benchmarks, run the applications in the respective examples. BenchmarkDotNet will automatically run the defined benchmarks with various settings, gather results, and generate a report.
Analyzing the Results
BenchmarkDotNet will generate detailed reports, including metrics such as Mean, Median, StandardDeviation, and more, for each benchmark method. Analyze the reports to identify performance differences between different implementations.
The key metrics might include:
- Mean Time: The average time taken by each method to perform the string comparison over multiple iterations.
- Median Time: The middle value of the time taken by each method, which represents the “typical” or “average” performance.
- Standard Deviation: A measure of the variability or spread in the results. Lower values indicate more consistent performance.
- Min Time: The minimum time taken by each method in any single iteration.
- Max Time: The maximum time taken by each method in any single iteration.
Conclusion
BenchmarkDotNet is an essential tool for C# .NET developers to perform reliable and accurate performance benchmarking. By using this library, developers can measure and compare the performance of different code implementations, identify bottlenecks, and optimize their applications for maximum efficiency. With its simple API and powerful analysis capabilities, BenchmarkDotNet significantly simplifies the process of performance benchmarking in C# .NET. Whether you want to compare mathematical operations or evaluate sorting algorithms, BenchmarkDotNet can help you make informed decisions to deliver high-performance applications.