Are you still refreshing your web pages to see new data? What if your apps could talk to users instantly — no reloads, no delays?
Welcome to the world of real-time web apps with Blazor Server and SignalR. If you’ve ever wanted to build responsive, interactive UIs using only C# — no JavaScript required — this post is your gateway.
Blazor Server + SignalR = Real-Time Magic
Blazor Server is a part of the Blazor framework that lets you write rich, dynamic web applications in C#. All the logic runs on the server, and the UI updates via a SignalR connection. This makes it a fantastic tool for real-time features like chats, dashboards, notifications, or any live data scenarios.
Here’s how it works:
- UI events on the browser are sent to the server.
- The server processes and responds with UI diffs via SignalR.
- The client renders updates instantly — no full reloads.
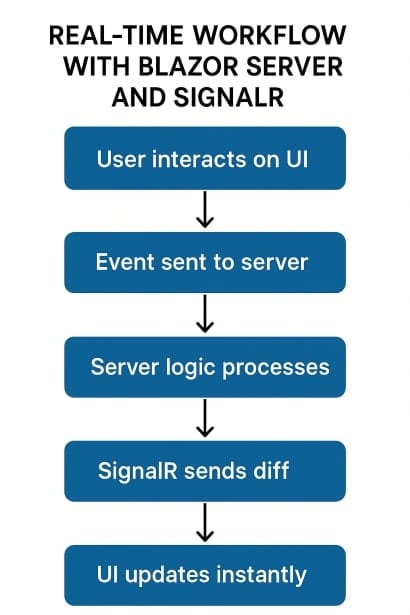
Step 1: Setting Up the Project
To create a new Blazor Server app, use the CLI:
dotnet new blazorserver -n RealTimeApp
Navigate to the new project folder and run it:
cd RealTimeApp
dotnet run
Now you’ve got a clean Blazor Server app running locally.
Step 2: Add SignalR Hub
Create a hub class in a new file ChatHub.cs
:
using Microsoft.AspNetCore.SignalR;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
Why this matters:
SignalR Hubs act as the central communication pipeline. In this example, SendMessage
sends data to all connected clients, which is what enables real-time updates.
Step 3: Configure SignalR in Startup.cs
Register SignalR in the services and configure endpoints:
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddServerSideBlazor();
services.AddSignalR();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseEndpoints(endpoints =>
{
endpoints.MapBlazorHub();
endpoints.MapHub<ChatHub>("/chathub");
endpoints.MapFallbackToPage("/_Host");
});
}
Explanation:
MapHub<ChatHub>("/chathub")
exposes your SignalR hub at /chathub
, which the frontend will use to connect and communicate.
Step 4: Create the Chat UI
Add this to a Blazor component like Pages/Chat.razor
:
@page "/chat"
@inject NavigationManager Navigation
<input @bind="userInput" placeholder="Name" />
<input @bind="messageInput" placeholder="Message" />
<button @onclick="Send">Send</button>
<ul>
@foreach (var msg in messages)
{
<li>@msg</li>
}
</ul>
@code {
private HubConnection? hubConnection;
private string? userInput;
private string? messageInput;
private List<string> messages = new();
protected override async Task OnInitializedAsync()
{
hubConnection = new HubConnectionBuilder()
.WithUrl(Navigation.ToAbsoluteUri("/chathub"))
.Build();
hubConnection.On<string, string>("ReceiveMessage", (user, message) =>
{
messages.Add($"{user}: {message}");
StateHasChanged();
});
await hubConnection.StartAsync();
}
private async Task Send()
{
if (hubConnection is not null)
{
await hubConnection.SendAsync("SendMessage", userInput, messageInput);
}
}
}
How it works:
- The
HubConnection
listens for new messages from the server. - When
Send
is called, the message is sent via SignalR. - All clients receive and render it — instantly.
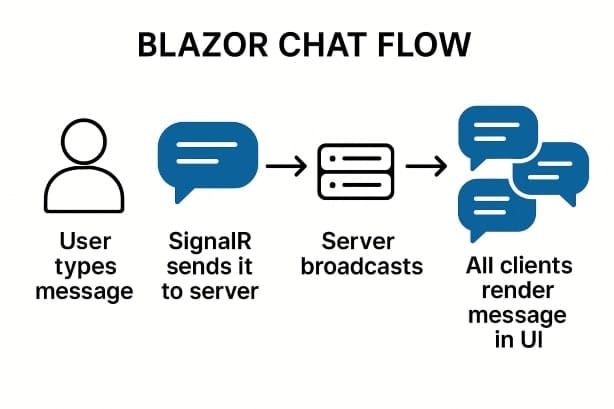
FAQ: Real-Time Blazor Development
Yes, but Blazor Server is easier to set up and works well for SignalR due to its persistent connection model.
SignalR automatically attempts to reconnect. You can hook into those events for custom UX.
Yes. In production, use Azure SignalR Service or a Redis backplane to handle scale-out scenarios.
Conclusion: Real-Time in Blazor is Easier Than You Think
Building real-time apps used to mean wrestling with JavaScript, polling, and external libraries. With Blazor and SignalR, you can create live, interactive UIs in pure C# with just a few lines of code.
Try adding SignalR to your next dashboard, notification center, or chat app — your users will feel the difference.
Have you tried real-time features in Blazor yet? Share your experience or questions in the comments!