Inheritance: Base and Derived Classes
Object-oriented C# programming (OOP) centrally features inheritance as one of its core concepts. Inheritance allows you to create a new class that is based on an existing class in C#. The existing class is known as the base class, while the new class is referred to as the derived class. The derived class inherits the members (e.g., fields, properties, and methods) of the base class, and it can also add new members or override the inherited ones.
Base Class
In C#, derived classes build upon the foundation that the base class provides. The base class may define certain methods and properties that are common to all its derived classes.
Example:
// This class represents a generic car with basic behaviors.
public class Car
{
// Property representing the model of the car.
public string Model { get; set; }
// Method for the car to "ride".
// When called, it outputs a message indicating the car is riding.
public void Ride()
{
Console.WriteLine($"{Model} is riding.");
}
}
Derived Class
The derived class inherits from the base class, meaning it takes on its members and can also add or modify them.
Example:
// The Jeep class inherits from the Car class.
// This means a Jeep is a specific type of Car with additional behaviors.
public class Jeep : Car
{
// Method for the jeep to "beep".
// When called, it outputs a message indicating the jeep is beeping.
public void Beep()
{
Console.WriteLine($"{Model} is beeping.");
}
}
In the example given above, the Jeep
class is based on the Car
class. This means that a Jeep
object can utilize the Ride
method from the Car
class and also the Beep
method that is specific to the Jeep
class.
Key Concepts
- Inheritance Chain: A derived class can serve as a base class for another class, creating a chain of inheritance. For example:
Car
->PassengerCar
->Jeep
. - Access Modifiers: Not all members of the base class are accessible in the derived class. It depends on their access modifiers:
public
: Accessible everywhere.protected
: Accessible in the base class and derived classes.private
: Not accessible outside the class in which they are declared.internal
: Accessible within the same assembly.
- Method Overriding: If the derived class needs a base class method to behave differently, it can override it. This requires the base class method to be marked with the
virtual
keyword, and the derived class method should use theoverride
keyword. Let’s see example:
public class Car
{
public virtual void MakeBeep()
{
Console.WriteLine("The car makes a sound.");
}
}
public class Jeep: Car
{
public override void MakeBeep()
{
Console.WriteLine("The Jeep beeps.");
}
}
- Base Keyword: It allows you to call a method or constructor in the base class from the derived class. Example:
public class Jeep: Car
{
public Jeep(string model)
{
// Use the base keyword to access the base class's property
base.Model = model;
}
}
- Sealed Classes: You can mark a class as sealed if you don’t want other classes to inherit from it.
Why Use Inheritance?
- Code Reusability: One of the primary benefits is that you don’t have to recreate methods or properties that already exist in the base class. This leads to less code duplication.
- Establish Relationship: Inheritance helps in establishing a “is-a” relationship between the base and derived classes. For instance, a
Jeep
“is-a”Car
. - Extensibility: It’s easier to add additional features or functionalities to a derived class without altering the base class.
Important Points about Inheritance in C#
- Single Inheritance: C# supports single inheritance, meaning a class cannot inherit from more than one class at a time. However, a class can implement multiple interfaces.
- Base Class Initialization: When creating an instance of a derived class, the constructor of the base class is called first. This process ensures proper initialization of the base class before the derived class begins its own initialization.
- Protected Access Modifier: The
protected
access modifier is vital in inheritance. Members marked asprotected
are accessible within their own class and by derived classes. This is especially useful when you want derived classes to have access to certain members of the base class but don’t want those members to be public. - Method Overriding: This is a crucial feature that allows a derived class to provide a specific implementation for a method already defined in its base class. The base class’s method needs to be marked with the
virtual
keyword, and the derived class should use theoverride
keyword. This enables polymorphism, allowing you to invoke the derived class’s method even when using a reference of the base class type. - Keyword
base
: Thebase
keyword in C# is used to call the base class’s members (like methods, properties, and constructors). It’s handy when you want to refer explicitly to the base class’s implementation, especially in overridden methods or derived class constructors. - Sealed Classes: If a class is marked as
sealed
, it cannot be inherited. You can use this to restrict a class from further extension, either for security reasons or design considerations. - Abstract Classes: You cannot instantiate an abstract class, and it might contain abstract members (methods, properties, etc.) lacking any implementation. Derived classes inheriting from an abstract class must provide an implementation for these abstract members. This is a way to ensure a certain “contract” or structure for derived classes.
Polymorphism and Overriding
Polymorphism and overriding are important concepts in object-oriented C# programming (OOP). They are crucial for creating flexible and reusable code. Polymorphism allows developers to design classes and objects with various forms and behaviors, depending on their usage. This promotes code reusability and makes software development more efficient. Overriding allows developers to change the behavior of inherited methods in a derived class, allowing customization and specialization. By learning about these concepts, programmers can understand how to use polymorphism and overriding to build strong and extensible software systems.
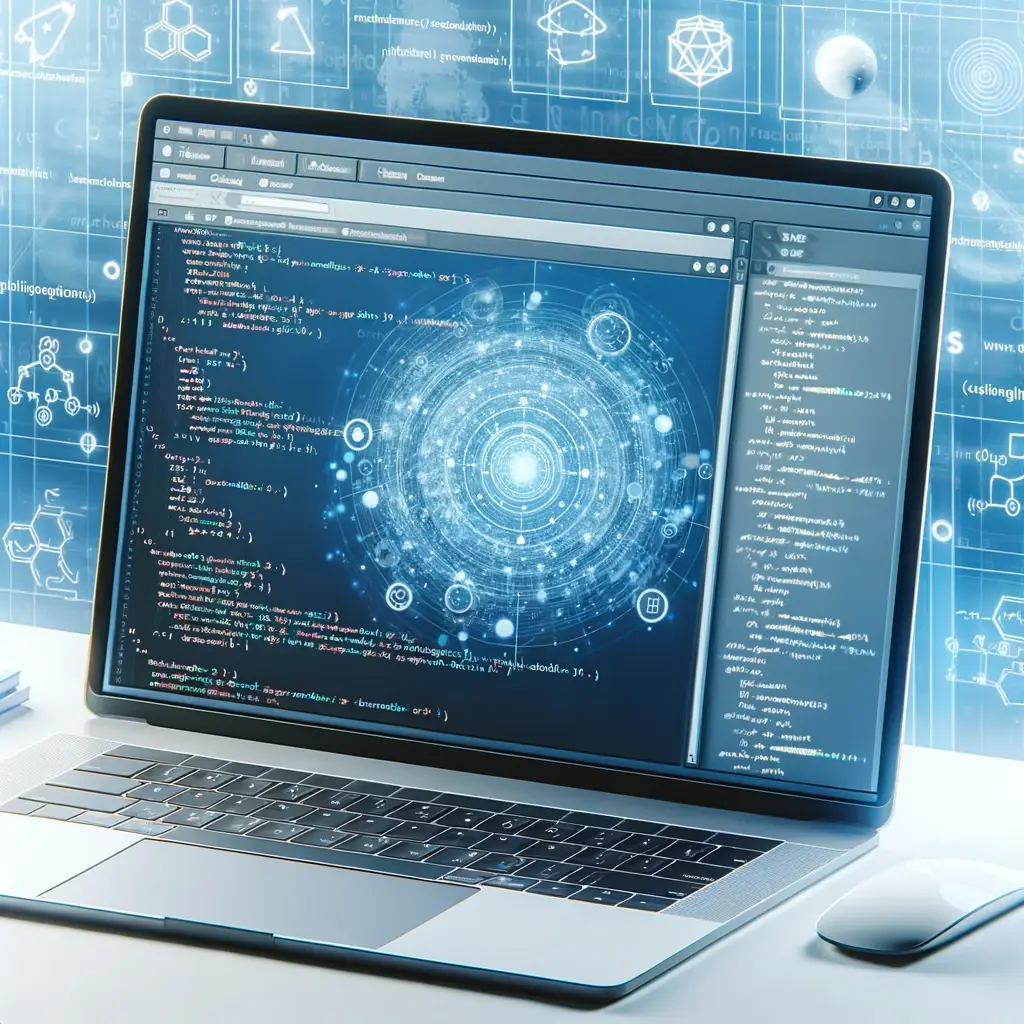
Polymorphism
Polymorphism enables treating objects of different classes as objects of a common superclass. The most common application of polymorphism occurs when a parent class reference refers to a child class object. This is especially beneficial in scenarios where we want to execute one action in different ways for different subclasses.
Two Types of Polymorphism in C#
- Compile-Time Polymorphism: This determination happens at compile time and is achieved through method overloading (using the same method name but with different signatures) or operator overloading (defining new behavior for standard operators for user-defined data types).
- Run-Time Polymorphism: This determination occurs during runtime. Method overriding, which involves redefining a base class’s method in a derived class, is a primary example of this.
Method Overriding
To achieve runtime polymorphism through method overriding, specific criteria must be met:
- Base class method must be marked with the
virtual
keyword. - Derived class method uses the
override
keyword. - Both the base and derived class methods must have the same name, return type, and parameters.
Why is Overriding Important?
Imagine you’re building a zoo management system. All animals eat, but they eat different foods in different ways. You’d have a base class Car
with a method Ride()
. Without polymorphism, you’d need separate procedures for every animal type. However, with polymorphism, you can simply override the Ride()
method for each animal type, allowing for more organized and scalable code.
Example:
// This is a base class representing a generic car.
public class Car
{
// A virtual method that can be overridden by derived classes.
// Specifies the general behavior of riding for a car.
public virtual void Ride()
{
Console.WriteLine("This car rides.");
}
}
// The Tesla class is derived from the Car base class.
// It represents a specific type of car with unique behaviors.
public class Tesla: Car
{
// Overrides the base class's Ride method.
// Specifies the tesla's specific riding behavior.
public override void Ride()
{
Console.WriteLine("Tesla drives on electricity.");
}
}
// The Jeep class is derived from the Car base class.
// It represents another specific type of car with distinct behaviors.
public class Jeep: Car
{
// Overrides the base class's Ride method.
// Specifies the jeep's specific ride behavior.
public override void Ride()
{
Console.WriteLine("Jeep drives on gasoline.");
}
}
Using the above classes:
Car myCar = new Tesla();
myCar.Ride(); // Outputs: "Tesla drives on electricity."
myCar = new Jeep();
myCar.Ride(); // Outputs: "Jeep drives on gasoline."
Here, even though the reference is of type Car
, the correct Ride
method associated with the actual object type (Tesla
or Jeep
) is called. This is runtime polymorphism in action.
Additional Points
- The
base
Keyword: You can use thebase
keyword in the derived class to call the method of the base class. This is useful when you want to extend (rather than replace) the base method’s behavior. - Abstract Methods: If a method in the base class is marked as
abstract
, the derived class MUST provide an implementation (override it). The base class cannot provide an implementation for an abstract method. - Sealed Overridden Methods: If you override a method and don’t want any further derived classes to override it again, you can mark the method with the
sealed
keyword.
Polymorphism and method overriding offer a way to design more generic and reusable classes in Object-Oriented Programming C#. They allow derived classes to have methods with the same names as the base class but with potentially different implementations. This enables more intuitive class designs and more readable, organized code structures. By understanding and leveraging these concepts, you can create more adaptable and maintainable software systems.
Abstract Classes and Interfaces
Both abstract classes and interfaces are important concepts in C# and object-oriented programming (OOP). They serve as a guide for other classes, making sure the code is consistent and easy to maintain. Primarily, developers use abstract classes to create a base class hierarchy that enables code reuse and inheritance. On the other hand, interfaces define behaviors that multiple classes can use, thus making the code flexible and enabling polymorphism. Knowing the differences between these two concepts is key to designing and structuring C# applications effectively.
Abstract Classes
Definition: In object-oriented programming with C#, an abstract class represents a special class type that you cannot create directly. Instead, its purpose is to serve as a blueprint or template from which other classes can inherit to gain its properties and behaviors. By offering a common set of attributes and methods, abstract classes allow for code reuse and encourage a more organized and modular software design.
Key Characteristics of Abstract Classes:
- Instantiation: You cannot create an instance of an abstract class directly.
- Members: Abstract classes can have fields, properties, methods, events, and indexers.
- Abstract Members: Abstract classes can include abstract methods or properties, which developers declare without implementing them. Any non-abstract derived class must provide an implementation for these members.
- Concrete Members: Apart from abstract members, an abstract class can also contain fully implemented methods, properties, etc.
- Access Modifiers: Abstract members can have access modifiers like
public
,protected
, etc.
Example of Abstract Class:
public abstract class Car
{
public string Color { get; set; }
public abstract void Beep(); // Abstract method
public void Ride() // Concrete method
{
Console.WriteLine("The car rides.");
}
}
Interfaces
Definition: An interface is a concept in object-oriented programming that allows classes or structs to agree to provide specific methods, properties, events, or indexers. By implementing an interface, a class can ensure consistent behavior across different implementations. Interfaces do not have any actual implementation themselves, but serve as a blueprint for the expected behavior of implementing classes.
Key Characteristics of Interfaces:
- Instantiation: Similar to abstract classes, you cannot instantiate interfaces directly.
- Members: Interfaces can have methods, properties, events, and indexers, but no fields (instance variables).
- No Implementation: Interface members are implicitly abstract and public. They cannot have any implementation.
- Multiple Inheritance: A class can implement multiple interfaces, overcoming the single inheritance limitation of classes in C#.
Example of Interface:
// This interface defines a contract for objects that can "beep".
public interface IBeep
{
// Method declaration for "Beep".
void Beep();
}
Differences & When to Use
- Inheritance vs. Implementation: Abstract classes are extended using the
extends
keyword. Interfaces are implemented using theimplements
keyword. - Multiple Inheritance: In C#, a class cannot inherit from more than one class (single inheritance). However, it can implement multiple interfaces.
- State: Abstract classes can have fields, while interfaces cannot.
- Access Modifiers: In abstract classes, you can have a variety of access modifiers for methods, but interface methods are always implicitly public and cannot have access modifiers.
When to Use Abstract Classes:
- When you want to provide a common base class for related classes.
- When you need to share code among several closely related classes (i.e., concrete methods).
- You declare non-public members, such as protected abstract members, when you want derived classes to implement them without making them publicly accessible.
When to Use Interfaces:
- When multiple classes share only method signatures without a common lineage.
- When you need to achieve multiple inheritances of type.
- When you want to establish a contract for classes, even across different class hierarchies.
Both abstract classes and interfaces allow you to define a blueprint for classes, but they serve different architectural purposes. Abstract classes offer a foundation for sharing implementation among closely related classes, whereas interfaces define a contract that any class can adopt, irrespective of its position in the class hierarchy. Knowing when to use each one effectively can greatly improve the design and maintainability of your software.
The sealed Keyword
In C#, the sealed keyword prevents inheritance of a class or overriding of a method. It essentially means you’ve created a class or method in its final form, and you don’t want any modifications through inheritance.
Why Use sealed?
- Control: You might lock down a class’s behavior or design to ensure other developers do not misuse or misinterpret it.
- Safety: To guarantee that the behavior of a class or method doesn’t change in subclasses, which might introduce unpredicted issues.
- Minor Performance Benefits: Sealed classes can sometimes offer marginal speed advantages due to optimizations by the JIT compiler.
Where Can You Use sealed?
On Classes: When you seal a class, no other class can inherit from it.
sealed class MyFinalCarClass
{
// class content
}
In the example above, no other class can inherit from MyFinalCarClass
.
On Overridden Methods in Derived Classes: You can seal a method overridden in a derived class to prevent any further derived classes from overriding it again.
public class BaseCarClass
{
public virtual void MyMethod() { }
}
public class ChildCarClass : BaseCarClass
{
public sealed override void MyMethod() { }
}
Here, any class inheriting from ChildCarClass
cannot override MyMethod
again.
Things to Remember
- Not For Every Class: It’s not necessary to seal every class. Use it when you have a specific reason to prevent inheritance.
- Can’t Seal Interface Methods: Interface methods are implicitly virtual, so you can’t seal them directly. However, when a class implements an interface method, you can seal that implementation.
The sealed
keyword plays a vital role in providing control over inheritance. It helps encapsulate the functionality and ensures that certain classes or methods remain in their defined state without further extensions or modifications. While a powerful tool for class design, you should use it judiciously, ensuring that it aligns with the software’s architectural goals.
FAQ
Inheritance is one of the fundamental pillars of Object-Oriented Programming (OOP) in C#. It allows a class (the derived class) to inherit members (like fields, methods, and events) from another class (the base class).
No, C# supports single inheritance, which means a derived class can inherit from only one base class. However, a class can implement multiple interfaces.
The base
keyword in C# is used to access members of the base class from a derived class. For instance, you can use base.MethodName()
to call a method in the base class that might have been overridden in the derived class.
You cannot override constructors as they lack the concept of virtuality. However, derived classes can call base class constructors using the base
keyword.
An interface defines a contract that classes or structs can implement. It can contain method, property, event, or indexer declarations without any implementation.
Yes, a class (or struct) can implement multiple interfaces, allowing for a form of multiple inheritance.
If a non-abstract class does not provide implementations for all members of an interface it implements, it will result in a compile-time error.
The sealed
keyword is a modifier in C# that prevents further inheritance of a class or overriding of a method.
Yes, you can use the sealed
keyword with methods in a derived class to prevent further derived classes from overriding that method.
Yes, but indirectly. The corresponding methods of properties (getters and setters), events (add and remove), and indexers can be sealed in a derived class if they were previously marked as virtual
or override
.